以直方图均衡理论基础,编程实现图像直方图匹配算法并还原成彩色
时间: 2023-08-04 12:14:52 浏览: 157
直方图匹配(Histogram Matching)是一种通过调整图像像素值分布来改善图像质量的方法,也称为直方图规定化(Histogram Specification)或直方图均衡化(Histogram Equalization)。
直方图匹配的核心思想是将待处理图像的像素值映射到一个新的像素值域,使得新的像素值域的像素值分布符合预期的分布,从而达到图像增强的目的。具体步骤如下:
1. 计算待处理图像和目标图像的直方图分布。
2. 对待处理图像的直方图进行归一化处理,得到累计分布函数。
3. 对目标图像的直方图进行归一化处理,得到累计分布函数。
4. 对待处理图像的每个像素值,找到目标图像累计分布函数最接近的像素值,将待处理图像像素值替换成目标图像像素值。
5. 对处理后的图像进行彩色还原。
下面是一个简单的Python程序,实现了灰度图像的直方图匹配和彩色还原功能:
```python
import cv2
import numpy as np
# 读取待处理图像和目标图像
src_img = cv2.imread('src_img.png', 0)
dst_img = cv2.imread('dst_img.png', 0)
# 计算直方图
src_hist, _ = np.histogram(src_img.flatten(), 256, [0, 256])
dst_hist, _ = np.histogram(dst_img.flatten(), 256, [0, 256])
# 计算累计分布函数
src_cdf = src_hist.cumsum()
dst_cdf = dst_hist.cumsum()
# 归一化累计分布函数
src_cdf_normalized = src_cdf * 255 / src_cdf[-1]
dst_cdf_normalized = dst_cdf * 255 / dst_cdf[-1]
# 直方图匹配
src_img_matched = np.interp(src_img.flatten(), range(0, 256), src_cdf_normalized).reshape(src_img.shape)
dst_img_matched = np.interp(dst_img.flatten(), range(0, 256), dst_cdf_normalized).reshape(dst_img.shape)
# 进行彩色还原
src_img_color = cv2.imread('src_img.png')
dst_img_color = cv2.imread('dst_img.png')
b, g, r = cv2.split(src_img_color)
src_img_color_matched = cv2.merge([src_img_matched, src_img_matched, src_img_matched])
dst_img_color_matched = cv2.merge([dst_img_matched, dst_img_matched, dst_img_matched])
# 显示结果
cv2.imshow('src_img_matched', src_img_matched)
cv2.imshow('dst_img_matched', dst_img_matched)
cv2.imshow('src_img_color_matched', src_img_color_matched)
cv2.imshow('dst_img_color_matched', dst_img_color_matched)
cv2.waitKey(0)
```
需要注意的是,彩色还原时需要将RGB三个通道分别进行直方图匹配,然后再合并成一张彩色图像。
阅读全文
相关推荐
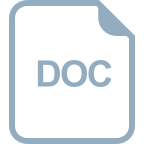
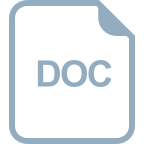
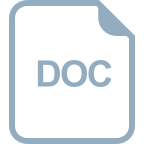
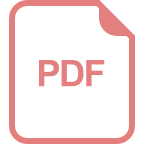
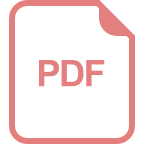
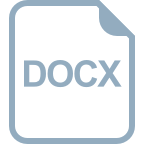
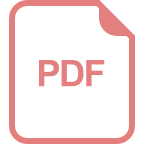
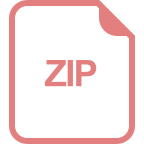
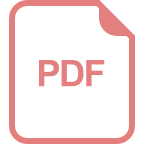
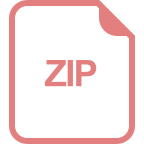
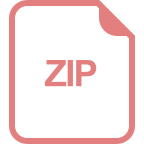
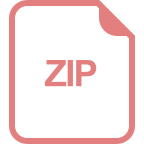
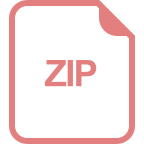
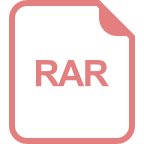
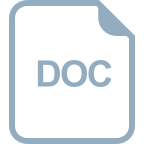
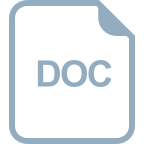
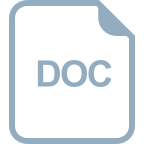
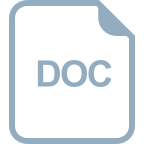
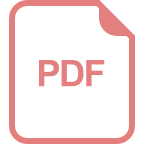
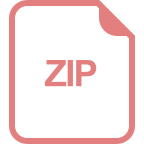