newName = ''.join((choice(ascii_letters) for i in range(n)))详解
时间: 2024-05-25 19:15:45 浏览: 45
这段代码使用了Python的内置函数`join()`和`choice()`,以及字符串常量`ascii_letters`。以下是对每个部分的详细解释:
- `choice(ascii_letters)`:`choice()`函数从给定的序列中随机选择一个元素并返回。`ascii_letters`是一个字符串常量,包含所有ASCII字符中的大小写字母。因此,`choice(ascii_letters)`会随机选择一个字母并返回。
- `(choice(ascii_letters) for i in range(n))`:这是一个Python生成器表达式,它将`choice(ascii_letters)`的结果重复n次,并将这些结果作为一个可迭代的对象。
- `''.join((choice(ascii_letters) for i in range(n)))`:`join()`函数将可迭代对象中的所有元素连接成一个字符串。在这里,我们将生成器表达式的结果作为可迭代对象,并使用空字符串`''`作为分隔符将所有元素连接起来。这样,我们就得到了一个长度为n的随机字母字符串。
因此,这段代码的作用是生成一个长度为n的随机字母字符串,并将其赋值给变量`newName`。
相关问题
将下面代码简洁化:def split_dataset(img_path, target_folder_path, output_path): filename = [] total_imgs = os.listdir(img_path) #for root, dirs, files in os.walk(img_path): for img in total_imgs: filename.append(img) np.random.shuffle(filename) train = filename[:int(len(filename) * 0.9)] test = filename[int(len(filename) * 0.9):] out_images = os.path.join(output_path, 'imgs') if not os.path.exists(out_images): os.makedirs(out_images) out_images_train = os.path.join(out_images, 'training') if not os.path.exists(out_images_train): os.makedirs(out_images_train) out_images_test = os.path.join(out_images, 'test') if not os.path.exists(out_images_test): os.makedirs(out_images_test) out_annotations = os.path.join(output_path, 'annotations') if not os.path.exists(out_annotations): os.makedirs(out_annotations) out_annotations_train = os.path.join(out_annotations, 'training') if not os.path.exists(out_annotations_train): os.makedirs(out_annotations_train) out_annotations_test = os.path.join(out_annotations, 'test') if not os.path.exists(out_annotations_test): os.makedirs(out_annotations_test) for i in train: print(os.path.join(img_path, i)) print(os.path.join(out_images_train, i)) shutil.copyfile(os.path.join(img_path, i), os.path.join(out_images_train, i)) annotations_name = "gt_" + i[:-3] + 'txt' shutil.copyfile(os.path.join(target_folder_path, annotations_name), os.path.join(out_annotations_train, annotations_name)) for i in test: shutil.copyfile(os.path.join(img_path, i), os.path.join(out_images_test, i)) annotations_name = "gt_" + i[:-3] + 'txt' shutil.copyfile(os.path.join(target_folder_path, annotations_name), os.path.join(out_annotations_test, annotations_name))
def split_dataset(img_path, target_folder_path, output_path):
filename = os.listdir(img_path)
np.random.shuffle(filename)
train = filename[:int(len(filename) * 0.9)]
test = filename[int(len(filename) * 0.9):]
out_images = os.path.join(output_path, 'imgs')
os.makedirs(out_images, exist_ok=True)
out_images_train = os.path.join(out_images, 'training')
os.makedirs(out_images_train, exist_ok=True)
out_images_test = os.path.join(out_images, 'test')
os.makedirs(out_images_test, exist_ok=True)
out_annotations = os.path.join(output_path, 'annotations')
os.makedirs(out_annotations, exist_ok=True)
out_annotations_train = os.path.join(out_annotations, 'training')
os.makedirs(out_annotations_train, exist_ok=True)
out_annotations_test = os.path.join(out_annotations, 'test')
os.makedirs(out_annotations_test, exist_ok=True)
for i in train:
img_src = os.path.join(img_path, i)
img_dst = os.path.join(out_images_train, i)
shutil.copyfile(img_src, img_dst)
annotations_name = "gt_" + i[:-3] + 'txt'
annotations_src = os.path.join(target_folder_path, annotations_name)
annotations_dst = os.path.join(out_annotations_train, annotations_name)
shutil.copyfile(annotations_src, annotations_dst)
for i in test:
img_src = os.path.join(img_path, i)
img_dst = os.path.join(out_images_test, i)
shutil.copyfile(img_src, img_dst)
annotations_name = "gt_" + i[:-3] + 'txt'
annotations_src = os.path.join(target_folder_path, annotations_name)
annotations_dst = os.path.join(out_annotations_test, annotations_name)
shutil.copyfile(annotations_src, annotations_dst)
解释下面代码:import os from config import paras para = paras() def revise_rawdata_name(para): op_dir = para.rawdata_dir save_dir = para.save_dir classes = os.listdir(op_dir) # 获取文档内所有文件 for class_name in classes: i = 1 con_dir = os.path.join(op_dir, class_name) save_path = os.path.join(save_dir, class_name) files = os.listdir(con_dir) #获取文档内所有文件 for file_name in files: # 取出路径父文件夹下各文件名 newname = f'{i:05}' (filename, extension) = os.path.splitext(file_name) str = filename.replace(filename,newname) os.rename(os.path.join(con_dir, file_name), os.path.join(save_path, str + extension)) # 添加前缀 i = i+1 def revise_name(para): ip_dir = para.rawdata_dir classes = os.listdir(ip_dir) # 获取文档内所有文件 for class_name in classes: con_dir = os.path.join(ip_dir, class_name) files = os.listdir(con_dir) #获取文档内所有文件 i = 0 for file_name in files: # 取出路径父文件夹下各文件名 (filename, extension) = os.path.splitext(file_name) newname = class_name +'_'+ str(i) os.rename(os.path.join(con_dir, file_name), os.path.join(con_dir, newname + extension)) # 添加前缀 i = i+1 # Press the green button in the gutter to run the script. if __name__ == '__main__': revise_name(para)
这段代码主要是用于修改文件名的函数。代码首先导入了`os`模块和一个名为`paras`的配置文件。然后定义了两个函数`revise_rawdata_name`和`revise_name`,并传入了一个参数`para`。
`revise_rawdata_name`函数的作用是将指定目录下的文件名进行修改,添加前缀。具体步骤如下:
1. 获取指定目录下的所有文件夹。
2. 遍历每个文件夹,获取文件夹路径。
3. 创建保存修改后文件的目录路径。
4. 获取当前文件夹下的所有文件。
5. 遍历每个文件,获取文件名和扩展名。
6. 根据计数变量`i`生成新的文件名。
7. 使用`os.rename`函数将原文件移动到新的保存目录,并添加前缀。
`revise_name`函数的作用是将指定目录下的文件名进行修改,添加前缀。具体步骤如下:
1. 获取指定目录下的所有文件夹。
2. 遍历每个文件夹,获取文件夹路径。
3. 获取当前文件夹下的所有文件。
4. 遍历每个文件,获取文件名和扩展名。
5. 根据文件夹的名称和计数变量`i`生成新的文件名。
6. 使用`os.rename`函数将原文件移动到同一目录,并添加前缀。
最后,在主程序中调用了`revise_name`函数,并传入了参数`para`来执行文件名修改操作。
阅读全文
相关推荐
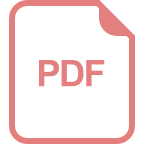
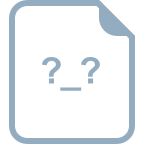
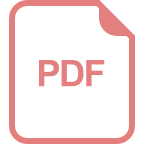














