PImage[] textures; float xRotation, yRotation; int mouseX, mouseY; void setup() { size(800, 600, P3D); textures = new PImage[5]; textures[0] = loadImage("texture1.jpg"); textures[1] = loadImage("texture2.jpg"); textures[2] = loadImage("texture3.jpg"); textures[3] = loadImage("texture4.jpg"); textures[4] = loadImage("texture5.jpg"); xRotation = 0; yRotation = 0; } void draw() { background(0); translate(width/2, height/2, 0); rotateX(radians(yRotation)); rotateY(radians(xRotation)); float spacing = 50; int numBoxesX = ceil(width/spacing); int numBoxesY = ceil(height/spacing); for (int i = 0; i < numBoxesX; i++) { for (int j = 0; j < numBoxesY; j++) { pushMatrix(); translate((i - numBoxesX/2) * spacing, (j - numBoxesY/2) * spacing, 0); texture(textures[int(random(textures.length))]); box(BOX_WIDTH, BOX_HEIGHT, BOX_WIDTH); popMatrix(); } } } void mouseMoved() { xRotation = map(mouseX, 0, width, 0, ROTATION_RANGE); yRotation = map(mouseY, 0, height, 0, ROTATION_RANGE); }的伪码
时间: 2024-04-06 19:33:31 浏览: 122
这段代码实现了使用Processing的P3D渲染器创建一个带有纹理的三维场景,其中包括多个立方体对象,每个立方体使用随机选择的一张纹理进行贴图,并且可以通过鼠标移动来改变场景中的视角。具体来说,代码实现了以下功能:
1. 加载5张纹理图片并存储在textures数组中。
2. 在setup()函数中初始化场景的旋转角度xRotation和yRotation。
3. 在draw()函数中设置背景颜色为黑色,并将坐标系的原点移动到屏幕中心。
4. 根据当前的旋转角度xRotation和yRotation分别绕x轴和y轴旋转整个场景。
5. 根据间距spacing和屏幕宽高计算出需要绘制的立方体的行列数numBoxesX和numBoxesY,并使用两个for循环分别遍历每个立方体的位置,然后将每个立方体的位置移动到对应的位置上。
6. 在每个立方体的位置上使用随机选择的一张纹理进行贴图,并绘制一个BOX_WIDTH、BOX_HEIGHT、BOX_WIDTH大小的立方体。
7. 在mouseMoved()函数中根据鼠标的位置映射出新的xRotation和yRotation,从而实现鼠标移动改变视角的效果。
注意,代码中的BOX_WIDTH和BOX_HEIGHT变量没有给出具体的值,需要根据实际需要进行设置。
相关问题
int CR_GetImage ( int nDetrIdx , void∗ pImage, int nImgSize) ;python调用为def函数
在Python中调用名为`CR_GetImage`的函数,可以使用以下代码示例:
```python
from ctypes import cdll, c_int, c_void_p
# 加载动态链接库
lib = cdll.LoadLibrary('your_library_path')
# 定义函数原型
lib.CR_GetImage.argtypes = [c_int, c_void_p, c_int]
lib.CR_GetImage.restype = c_int
# 调用函数
def CR_GetImage(nDetrIdx, pImage, nImgSize):
result = lib.CR_GetImage(nDetrIdx, pImage, nImgSize)
return result
```
请注意,这里的`your_library_path`应该替换为包含`CR_GetImage`函数的动态链接库的实际路径。另外,你可能需要根据实际情况修改函数原型的参数和返回类型。在这个例子中,`nDetrIdx`是整数类型的参数,`pImage`是指向图像数据的指针,`nImgSize`是图像数据的大小(以字节为单位)。函数返回一个整数结果。
import processing.serial.*; Serial myPort; PImage ocean = new PImage(); void setup() { myPort = new Serial(this, "COM3", 9600); size(1280,720); ocean = loadImage("ocean.jpg"); ocean.resize(width, height); } void draw() { image(ocean, 0, 0); loadPixels(); ocean.loadPixels(); while (myPort.available() > 0) { char inByte = myPort.readChar(); println(inByte); switch(inByte){ case'1': for (int x=0; x<width-1; x++) { for (int y=0; y<height; y++) { int loc1 = x+y*width; int loc2 = (x+1)+y*width; float b1 = brightness(ocean.pixels[loc1]); float b2 = brightness(ocean.pixels[loc2]); float diff = abs(b1-b2); if (diff>15) { pixels[loc1] =color(0); } else { pixels[loc1] =color(255); } } updatePixels(); } break; } } }这段代码有什么问题
这段代码看起来没有明显的语法错误,但是有一些问题需要注意:
1. 在 `for` 循环中,y 的循环次数应该是 `height-1`,否则会出现数组越界的问题;
2. 在 `for` 循环中,x 的循环次数应该是 `width-1`,否则会出现数组越界的问题;
3. 在 `for` 循环中,应该先对 `ocean` 图像进行像素值的比较,再根据比较结果修改 `pixels` 数组中对应像素的颜色值;
4. 在 `case` 语句中,如果没有匹配到任何条件,应该添加一个 `default` 分支,以避免出现未知错误。
下面是修改后的代码:
```java
import processing.serial.*;
Serial myPort;
PImage ocean = new PImage();
void setup() {
myPort = new Serial(this, "COM3", 9600);
size(1280, 720);
ocean = loadImage("ocean.jpg");
ocean.resize(width, height);
}
void draw() {
image(ocean, 0, 0);
loadPixels();
ocean.loadPixels();
while (myPort.available() > 0) {
char inByte = myPort.readChar();
println(inByte);
switch (inByte) {
case '1':
for (int x = 0; x < width - 1; x++) {
for (int y = 0; y < height - 1; y++) {
int loc1 = x + y * width;
int loc2 = (x + 1) + y * width;
float b1 = brightness(ocean.pixels[loc1]);
float b2 = brightness(ocean.pixels[loc2]);
float diff = abs(b1 - b2);
if (diff > 15) {
pixels[loc1] = color(0);
} else {
pixels[loc1] = color(255);
}
}
}
updatePixels();
break;
default:
// 如果没有匹配到任何条件,则执行该语句块
break;
}
}
}
```
请注意,这只是一个简单的示例代码,具体实现方式可能需要根据你的项目需求进行修改。
阅读全文
相关推荐
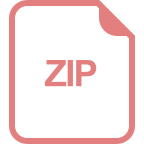
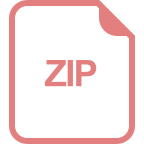
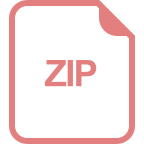
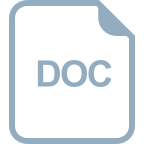












