用Python将一个Excel中的两列数据进行排列组合,然后将所有的组合结果追加合并写成另一列
时间: 2024-05-12 13:12:50 浏览: 179
可以使用Python中的pandas库来读取和处理Excel文件,具体实现可以按照以下步骤进行:
1. 安装pandas库:在命令行中输入`pip install pandas`即可安装。
2. 读取Excel文件:使用pandas库中的read_excel()函数读取Excel文件,例如:
```python
import pandas as pd
df = pd.read_excel('data.xlsx', sheet_name='Sheet1')
```
这里假设Excel文件名为data.xlsx,要处理的工作表名为Sheet1。
3. 排列组合:使用itertools库中的product()函数进行排列组合,例如:
```python
from itertools import product
result = list(product(df['列1'], df['列2']))
```
这里假设要对Excel文件中的“列1”和“列2”进行排列组合。
4. 追加合并:将排列组合的结果追加合并到Excel文件中的新列中,例如:
```python
df['组合结果'] = result
df.to_excel('output.xlsx', sheet_name='Sheet1', index=False)
```
这里将排列组合的结果存储在名为“组合结果”的新列中,然后将数据写入到名为“output.xlsx”的Excel文件中的“Sheet1”工作表中。
完整代码示例:
```python
import pandas as pd
from itertools import product
df = pd.read_excel('data.xlsx', sheet_name='Sheet1')
result = list(product(df['列1'], df['列2']))
df['组合结果'] = result
df.to_excel('output.xlsx', sheet_name='Sheet1', index=False)
```
注意:如果Excel文件中的数据量较大,使用product()函数会占用较多的内存,需要谨慎使用。此外,如果排列组合的结果需要去重,可以使用set()函数进行去重处理。
阅读全文
相关推荐
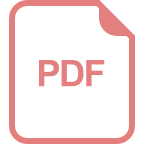
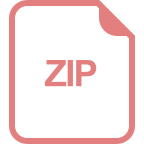




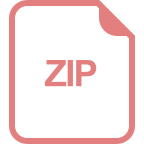
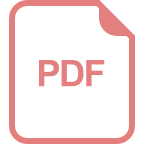
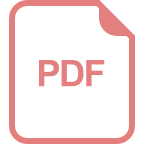
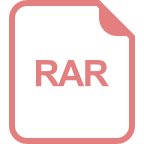
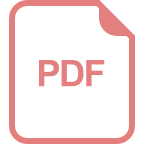
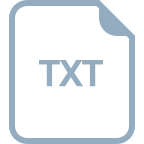
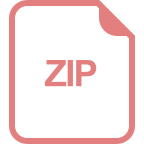