java银行家算法代码
时间: 2023-10-16 18:47:14 浏览: 100
当然,下面是一个简单的Java实现的银行家算法代码示例:
```java
import java.util.Arrays;
public class BankerAlgorithm {
private int processCount; // 进程数
private int resourceCount; // 资源种类数
private int[] available; // 可用资源数组
private int[][] max; // 最大需求矩阵
private int[][] allocation; // 分配矩阵
private int[][] need; // 需求矩阵
public BankerAlgorithm(int processCount, int resourceCount) {
this.processCount = processCount;
this.resourceCount = resourceCount;
this.available = new int[resourceCount];
this.max = new int[processCount][resourceCount];
this.allocation = new int[processCount][resourceCount];
this.need = new int[processCount][resourceCount];
}
public void setAvailable(int[] available) {
this.available = available;
}
public void setMax(int processIndex, int[] max) {
this.max[processIndex] = max;
}
public void setAllocation(int processIndex, int[] allocation) {
this.allocation[processIndex] = allocation;
}
public boolean isSafeState() {
boolean[] finish = new boolean[processCount];
int[] work = Arrays.copyOf(available, resourceCount);
int[][] tempNeed = new int[processCount][resourceCount];
int[] safeSequence = new int[processCount];
int count = 0;
for (int i = 0; i < processCount; i++) {
tempNeed[i] = Arrays.copyOf(need[i], resourceCount);
}
while (count < processCount) {
boolean found = false;
for (int i = 0; i < processCount; i++) {
if (!finish[i] && isLessOrEqual(tempNeed[i], work)) {
for (int j = 0; j < resourceCount; j++) {
work[j] += allocation[i][j];
}
safeSequence[count++] = i;
finish[i] = true;
found = true;
}
}
if (!found) {
break;
}
}
return count == processCount;
}
private boolean isLessOrEqual(int[] a, int[] b) {
for (int i = 0; i < a.length; i++) {
if (a[i] > b[i]) {
return false;
}
}
return true;
}
public static void main(String[] args) {
BankerAlgorithm banker = new BankerAlgorithm(5, 3);
int[] available = {3, 3, 2};
banker.setAvailable(available);
int[][] max = {
{7, 5, 3},
{3, 2, 2},
{9, 0, 2},
{2, 2, 2},
{4, 3, 3}
};
for (int i = 0; i < banker.processCount; i++) {
banker.setMax(i, max[i]);
}
int[][] allocation = {
{0, 1, 0},
{2, 0, 0},
{3, 0, 2},
{2, 1, 1},
{0, 0, 2}
};
for (int i = 0; i < banker.processCount; i++) {
banker.setAllocation(i, allocation[i]);
}
int[][] need = new int[banker.processCount][banker.resourceCount];
for (int i = 0; i < banker.processCount; i++) {
for (int j = 0; j < banker.resourceCount; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
boolean isSafe = banker.isSafeState();
System.out.println("Is the state safe? " + isSafe);
}
}
```
这个示例实现了银行家算法的安全性检查,通过判断系统是否处于安全状态来决定是否分配资源。在示例中,设置了5个进程和3个资源种类,分别为可用资源数组、最大需求矩阵、分配矩阵和需求矩阵。在 `main()` 方法中,设置了示例数据,并通过 `isSafeState()` 方法判断系统是否处于安全状态。
阅读全文
相关推荐
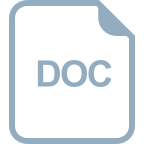
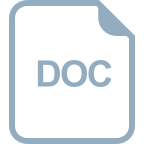
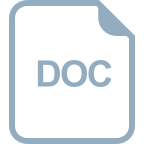




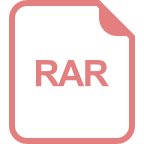
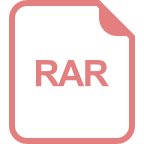
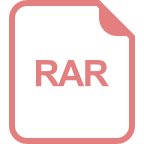
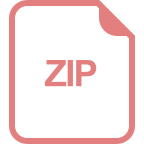
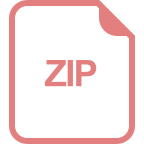
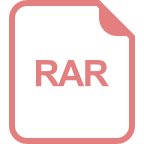
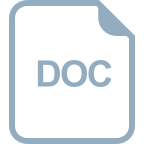
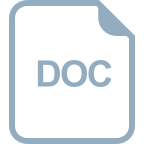

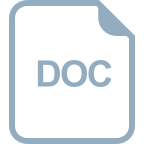