Java 银行家算法代码示例
时间: 2023-10-17 11:31:49 浏览: 82
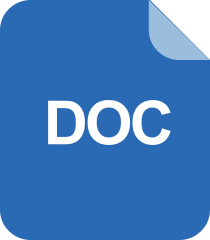
银行家算法实例

当然,下面是一个简单的Java代码示例,展了如何实现银行家算法:
```java
import java.util.Arrays;
public class BankerAlgorithm {
private int numOfProcesses; // 进程数量
private int numOfResources; // 资源数量
private int[] available; // 可用资源数
private int[][] max; // 最大需求矩阵
private int[][] allocation; // 已分配资源矩阵
private int[][] need; // 需求矩阵
public BankerAlgorithm(int numOfProcesses, int numOfResources, int[] available, int[][] max, int[][] allocation) {
this.numOfProcesses = numOfProcesses;
this.numOfResources = numOfResources;
this.available = available;
this.max = max;
this.allocation = allocation;
this.need = new int[numOfProcesses][numOfResources];
// 计算需求矩阵
for (int i = 0; i < numOfProcesses; i++) {
for (int j = 0; j < numOfResources; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
}
public boolean isSafeState() {
boolean[] finish = new boolean[numOfProcesses]; // 是否完成标记数组
int[] work = Arrays.copyOf(available, available.length); // 拷贝可用资源数组
// 初始化所有进程为未完成状态
Arrays.fill(finish, false);
// 寻找满足条件的进程分配资源,直到找不到满足条件的进程
while (true) {
int process = findNextProcess(finish, work);
if (process == -1) {
break;
}
// 标记当前进程为已完成
finish[process] = true;
// 释放当前进程已占用资源
for (int i = 0; i < numOfResources; i++) {
work[i] += allocation[process][i];
}
}
// 检查是否所有进程都已完成
for (boolean f : finish) {
if (!f) {
return false;
}
}
return true;
}
private int findNextProcess(boolean[] finish, int[] work) {
for (int i = 0; i < numOfProcesses; i++) {
// 检查进程是否已完成
if (finish[i]) {
continue;
}
// 检查进程是否满足资源需求
boolean canAllocate = true;
for (int j = 0; j < numOfResources; j++) {
if (need[i][j] > work[j]) {
canAllocate = false;
break;
}
}
if (canAllocate) {
return i;
}
}
return -1; // 未找到满足条件的进程
}
public static void main(String[] args) {
int numOfProcesses = 5;
int numOfResources = 3;
int[] available = {3, 3, 2};
int[][] max = {
{7, 5, 3},
{3, 2, 2},
{9, 0, 2},
{2, 2, 2},
{4, 3, 3}
};
int[][] allocation = {
{0, 1, 0},
{2, 0, 0},
{3, 0, 2},
{2, 1, 1},
{0, 0, 2}
};
BankerAlgorithm banker = new BankerAlgorithm(numOfProcesses, numOfResources, available, max, allocation);
if (banker.isSafeState()) {
System.out.println("当前状态是安全的");
} else {
System.out.println("当前状态是不安全的");
}
}
}
```
这个示例中,我们先初始化了进程数量、资源数量、可用资源数、最大需求矩阵和已分配资源矩阵等参数。然后通过计算得到需求矩阵。接下来,我们实现了`isSafeState()`方法来判断当前状态是否是安全的。
在主函数部分,我们提供了一个简单的例子,并调用银行家算法来判断当前状态是否是安全的。你可以根据需要修改输入参数和数据来进行测试。
注意:这只是一个简单的银行家算法示例,实际应用中可能需要更复杂的实现和处理异常情况。
阅读全文
相关推荐
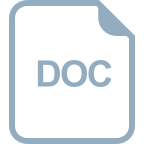

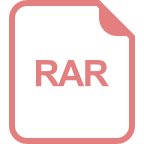
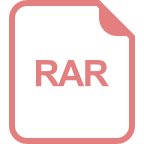
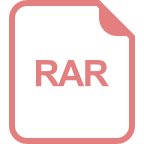
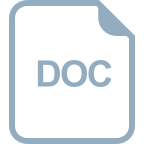
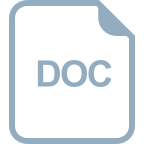
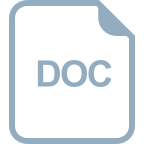







