#include<iostream> #include<vector> using namespace std; template<typename T> void print1(T &container) { for (int i = 0;i <container.size(); i++) { cout << container[i] << " "; ; } cout << endl; } int main() { int a[5] = { 1,2,3,4,5 }; vector<int> b = { 1,2,3,4,5 }; cout << "输出a" << std::endl; print1(a); cout << "输出b" << std::endl; print1(b); }修改正确
时间: 2024-03-06 18:50:05 浏览: 28
您好,根据您的代码,需要做以下修改才能正确输出:
1.将函数print1()中的for循环的循环变量i改为unsigned或者size_t类型,以避免i小于0的情况。
2.将函数print1()中的循环体中多余的分号去掉。
修改后的代码如下:
```
#include<iostream>
#include<vector>
using namespace std;
template<typename T>
void print1(T &container)
{
for (unsigned i = 0; i < container.size(); i++)
{
cout << container[i] << " ";
}
cout << endl;
}
int main()
{
int a[5] = { 1,2,3,4,5 };
vector<int> b = { 1,2,3,4,5 };
cout << "输出a" << std::endl;
print1(a);
cout << "输出b" << std::endl;
print1(b);
return 0;
}
```
这样就可以正确输出数组a和向量b的元素了。
相关问题
#include <iostream> #include <string> #include <stack> #include <vector> #include <iomanip> using namespace std; 逐行注释以上代码
这是一段C++的程序代码,使用了几个标准库的头文件。以下是逐行的注释:
```
#include <iostream> // 输入输出流库头文件
#include <string> // 字符串库头文件
#include <stack> // 栈库头文件
#include <vector> // 向量库头文件
#include <iomanip> // 格式控制库头文件
using namespace std; // 命名空间
```
这段程序代码导入了五个标准库的头文件,分别是iostream、string、stack、vector、iomanip,它们分别用于处理输入输出、字符串、栈、向量和格式控制。其中iostream是C++中最常用的输入输出流库,string是用于字符串操作的库,stack是用于栈数据结构的库,vector是用于动态数组的库,iomanip是用于格式化输出的库。
最后一行代码使用了命名空间std,这意味着所有的标准库函数和变量都在std命名空间中。如果不使用using namespace std,则需要在使用标准库函数时加上std::前缀。
#include <iostream> #include<algorithm> #include<cmath> #include <queue> using namespace std;
#include <iostream>:这是C++标准库中的头文件,用于输入输出流操作,包括cin、cout等。
#include<algorithm>:这是C++标准库中的头文件,用于提供各种算法操作,如排序、查找等。
#include<cmath>:这是C++标准库中的头文件,用于提供数学函数操作,如绝对值、平方根等。
#include <queue>:这是C++标准库中的头文件,用于提供队列操作,包括入队、出队等。
using namespace std;:这是C++的命名空间声明,表示使用std命名空间,可以直接使用std中的函数和对象。
相关推荐
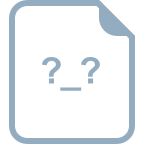
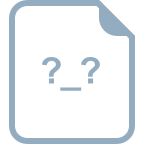













