用c语言编写 给定程序的功能是,将仅在字符串 s 中出现而不在字符串 t 中出现的字符,和仅在 t 中出现而不在字符串 s 中出现的字符,构成一个新的字符串放在 u 中,u 中字符按原字符串 中字符的顺序排列,不去掉重复字符
时间: 2023-06-18 21:04:52 浏览: 79
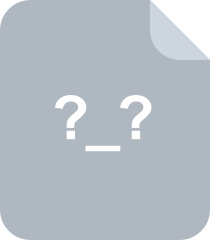
C语言程序设计-将字符串中的小写字母转换为对应的大写字母,其它字符不变;.c

以下是用 C 语言编写的实现代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
void find_unique_chars(char s[], char t[], char u[]) {
int freq_s[256] = {0}; // 记录 s 中字符出现的次数
int freq_t[256] = {0}; // 记录 t 中字符出现的次数
int len_s = strlen(s);
int len_t = strlen(t);
int i;
// 统计 s 中字符的出现次数
for (i = 0; i < len_s; i++) {
freq_s[s[i]]++;
}
// 统计 t 中字符的出现次数
for (i = 0; i < len_t; i++) {
freq_t[t[i]]++;
}
// 将仅在 s 中出现而不在 t 中出现的字符添加到 u 中
for (i = 0; i < len_s; i++) {
if (freq_s[s[i]] > 0 && freq_t[s[i]] == 0) {
u[strlen(u)] = s[i];
}
}
// 将仅在 t 中出现而不在 s 中出现的字符添加到 u 中
for (i = 0; i < len_t; i++) {
if (freq_t[t[i]] > 0 && freq_s[t[i]] == 0) {
u[strlen(u)] = t[i];
}
}
}
int main() {
char s[MAX_LEN], t[MAX_LEN], u[MAX_LEN];
printf("请输入字符串 s:");
gets(s); // 读入字符串 s
printf("请输入字符串 t:");
gets(t); // 读入字符串 t
find_unique_chars(s, t, u); // 查找唯一字符并存储到 u 中
printf("唯一字符序列为:%s\n", u);
return 0;
}
```
以上代码使用了三个字符数组 s、t 和 u 来存储输入的字符串和最终的结果,数组 u 最初为空,函数 `find_unique_chars` 用来查找唯一字符并将它们添加到数组 u 中。在函数中,我们首先用两个计数器数组 `freq_s` 和 `freq_t` 来记录字符在 s 和 t 中出现的次数,然后遍历 s 和 t 中的字符,将仅在 s 中出现而不在 t 中出现的字符和仅在 t 中出现而不在 s 中出现的字符添加到 u 中。最后,我们将 u 中的唯一字符序列输出到屏幕上。
注意,以上代码中输入字符串的读入函数 `gets` 已经被标记为不安全,请自行使用 `fgets` 等安全的字符串读入函数进行替换。
阅读全文
相关推荐
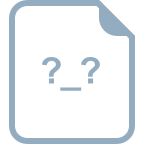
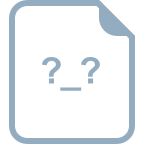




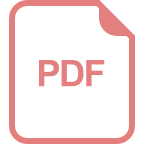
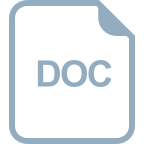








