java定义shape
时间: 2023-10-20 09:19:58 浏览: 53
在 Java 中,可以使用抽象类和接口来定义形状,如下所示:
```java
// 抽象类 Shape,定义了形状的基本属性和方法
abstract class Shape {
protected String color;
protected boolean filled;
public Shape() {
color = "red";
filled = true;
}
public Shape(String color, boolean filled) {
this.color = color;
this.filled = filled;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public boolean isFilled() {
return filled;
}
public void setFilled(boolean filled) {
this.filled = filled;
}
public abstract double getArea();
public abstract double getPerimeter();
@Override
public String toString() {
return "Shape{" +
"color='" + color + '\'' +
", filled=" + filled +
'}';
}
}
// 接口 Drawable,定义了形状的可绘制方法
interface Drawable {
void draw();
}
// 具体类 Circle,继承自 Shape,实现了 Drawable 接口
class Circle extends Shape implements Drawable {
protected double radius;
public Circle() {
radius = 1.0;
}
public Circle(double radius) {
this.radius = radius;
}
public Circle(double radius, String color, boolean filled) {
super(color, filled);
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public double getPerimeter() {
return 2 * Math.PI * radius;
}
@Override
public void draw() {
System.out.println("Drawing Circle");
}
@Override
public String toString() {
return "Circle{" +
"radius=" + radius +
", color='" + color + '\'' +
", filled=" + filled +
'}';
}
}
// 具体类 Rectangle,继承自 Shape,实现了 Drawable 接口
class Rectangle extends Shape implements Drawable {
protected double width;
protected double length;
public Rectangle() {
width = 1.0;
length = 1.0;
}
public Rectangle(double width, double length) {
this.width = width;
this.length = length;
}
public Rectangle(double width, double length, String color, boolean filled) {
super(color, filled);
this.width = width;
this.length = length;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
@Override
public double getArea() {
return width * length;
}
@Override
public double getPerimeter() {
return 2 * (width + length);
}
@Override
public void draw() {
System.out.println("Drawing Rectangle");
}
@Override
public String toString() {
return "Rectangle{" +
"width=" + width +
", length=" + length +
", color='" + color + '\'' +
", filled=" + filled +
'}';
}
}
```
在上述代码中,抽象类 `Shape` 定义了形状的基本属性和方法,如颜色、是否填充、获取面积、获取周长等。接口 `Drawable` 定义了形状的可绘制方法 `draw()`。具体类 `Circle` 和 `Rectangle` 继承自 `Shape`,并实现了 `Drawable` 接口,分别实现了自己的属性和方法。
相关推荐
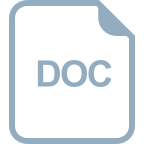
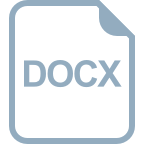














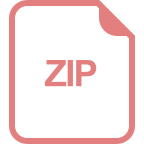