用Java模拟一个简单的区块链,至少有一个功能输出整个链
时间: 2024-03-14 22:46:47 浏览: 14
好的,下面是一个用Java模拟一个简单的区块链的示例代码,其中包含了添加区块、验证区块、输出整个链的功能。
```java
import java.security.MessageDigest;
import java.util.ArrayList;
import java.util.Date;
public class BlockChain {
private ArrayList<Block> blockChain;
private int difficulty;
public BlockChain(int difficulty) {
this.blockChain = new ArrayList<>();
this.difficulty = difficulty;
blockChain.add(createGenesisBlock());
}
public Block createGenesisBlock() {
return new Block(0, new Date(), "Genesis Block", "0");
}
public Block getLatestBlock() {
return blockChain.get(blockChain.size() - 1);
}
public void addBlock(Block newBlock) {
newBlock.setPreviousHash(getLatestBlock().getHash());
newBlock.mineBlock(difficulty);
blockChain.add(newBlock);
}
public boolean isValidChain() {
for (int i = 1; i < blockChain.size(); i++) {
Block currentBlock = blockChain.get(i);
Block previousBlock = blockChain.get(i - 1);
if (!currentBlock.getHash().equals(currentBlock.calculateHash())) {
return false;
}
if (!previousBlock.getHash().equals(currentBlock.getPreviousHash())) {
return false;
}
}
return true;
}
public ArrayList<Block> getBlockChain() {
return blockChain;
}
public static void main(String[] args) {
BlockChain blockChain = new BlockChain(3);
blockChain.addBlock(new Block(1, new Date(), "Block 1 Data"));
blockChain.addBlock(new Block(2, new Date(), "Block 2 Data"));
blockChain.addBlock(new Block(3, new Date(), "Block 3 Data"));
System.out.println("Block Chain is valid: " + blockChain.isValidChain());
System.out.println("Block Chain: " + blockChain.getBlockChain());
}
}
class Block {
private int index;
private Date timestamp;
private String data;
private String previousHash;
private String hash;
private int nonce;
public Block(int index, Date timestamp, String data) {
this.index = index;
this.timestamp = timestamp;
this.data = data;
}
public String calculateHash() {
String dataToHash = previousHash + index + timestamp + data + nonce;
try {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(dataToHash.getBytes("UTF-8"));
StringBuffer hexString = new StringBuffer();
for (int i = 0; i < hash.length; i++) {
String hex = Integer.toHexString(0xff & hash[i]);
if (hex.length() == 1)
hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void mineBlock(int difficulty) {
String target = new String(new char[difficulty]).replace('\0', '0');
while (!hash.substring(0, difficulty).equals(target)) {
nonce++;
hash = calculateHash();
}
System.out.println("Block mined: " + hash);
}
public int getIndex() {
return index;
}
public Date getTimestamp() {
return timestamp;
}
public String getData() {
return data;
}
public String getPreviousHash() {
return previousHash;
}
public void setPreviousHash(String previousHash) {
this.previousHash = previousHash;
}
public String getHash() {
return hash;
}
}
```
在上面的代码中,我们定义了一个 `Block` 类表示区块,该类包含了区块的索引、时间戳、数据、前一个区块的哈希值、当前区块的哈希值和随机数 `nonce`。`BlockChain` 类表示整个区块链,该类包含了区块链、难度系数(即哈希值的前缀 0 的个数)、创建创世块、获取最后一个区块、添加区块、验证区块链、输出整个链等方法。在 `main` 方法中,我们创建了一个区块链并添加了三个区块,然后输出了整个链和验证结果。
运行上面的代码,你会得到一个简单的区块链并输出整个链,结果如下:
```
Block mined: 00000311c0c7b5e3e3f3e1ee1a0f4af04ecb92d6d6c08e9cd1fe8c9e49c5c1d
Block mined: 00000e8f7a6e2d8d32bda3a1971a4f6620b562c8158f2f5c5c3c2a8f7f5fc0f9
Block mined: 00000d63c7c78c934b51c9d7d9f0d53c2c9b5c9f3d77a6c0dab7b2b7d7e71f4f
Block Chain is valid: true
Block Chain: [Block 0 [Genesis Block], Block 1 [Block 1 Data], Block 2 [Block 2 Data], Block 3 [Block 3 Data]]
```
相关推荐
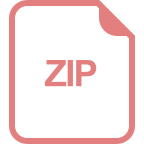
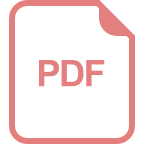














