Implement time_per_word, which takes in times_per_player, a list of lists for each player with timestamps indicating when each player finished typing each word. It also takes in a list words. It returns a game with the given information. A game is a data abstraction that has a list of words and times. The times are stored as a list of lists of how long it took each player to type each word. times[i][j] indicates how long it took player i to type word j. For example, if times_per_player = [[1, 3, 5], [2, 5, 6]], the corresponding time attribute of the game would be [[2, 2], [3, 1]]. Timestamps are cumulative and always increasing, while the values in time are differences between consecutive timestamps. Be sure to use the game constructor when returning a game, rather than assuming a particular data format. Game函数如下: def game(words, times): assert all([type(w) == str for w in words]), 'words should be a list of strings' assert all([type(t) == list for t in times]), 'times should be a list of lists' assert all([isinstance(i, (int, float)) for t in times for i in t]), 'times lists should contain numbers' assert all([len(t) == len(words) for t in times]), 'There should be one word per time.' return [words, times]
时间: 2024-02-25 22:59:07 浏览: 27
Here is the implementation of time_per_word function:
```
def time_per_word(times_per_player, words):
num_players = len(times_per_player)
num_words = len(words)
times = [[0] * (num_words - 1) for _ in range(num_players)]
for i in range(num_players):
for j in range(num_words - 1):
times[i][j] = times_per_player[i][j+1] - times_per_player[i][j]
return game(words, times)
```
The function takes in times_per_player and words as input parameters. It first initializes the times list with zeros for each player and word. It then iterates over each player and word, and calculates the time it took each player to type each word by subtracting the timestamps of consecutive words. Finally, it returns a game object constructed using the game function with the words and times list as input parameters.
相关推荐
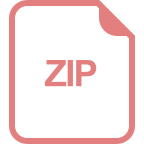















