用python实现去停用词
时间: 2023-05-20 13:03:23 浏览: 106
可以使用NLTK库来实现去停用词的功能,以下是示例代码:
```python
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
nltk.download('stopwords')
nltk.download('punkt')
stop_words = set(stopwords.words('english'))
def remove_stopwords(text):
word_tokens = word_tokenize(text)
filtered_text = [word for word in word_tokens if word.lower() not in stop_words]
return ' '.join(filtered_text)
```
在上面的代码中,我们首先导入了NLTK库和需要使用的模块,然后下载了英文停用词和分词器所需的数据。接着,我们定义了一个函数remove_stopwords,它接受一个字符串作为输入,并返回去除停用词后的字符串。在函数中,我们首先使用NLTK的word_tokenize函数将输入的字符串分词,然后使用列表推导式过滤掉其中的停用词,最后将过滤后的词重新组合成一个字符串并返回。
如果你想去除中文停用词,可以使用jieba库来实现。
相关问题
python去停用词-python使用jieba实现中文分词去停用词方法示例
好的,下面是python使用jieba实现中文分词去停用词的示例代码:
```python
import jieba
import jieba.analyse
# 加载自定义停用词表
jieba.analyse.set_stop_words("stopwords.txt")
# 待分词文本
text = "我喜欢学习自然语言处理"
# 中文分词
seg_list = jieba.cut(text)
# 去除停用词
final_list = []
for word in seg_list:
if word not in jieba.analyse.get_stop_words():
final_list.append(word)
# 输出结果
print(final_list)
```
其中,`stopwords.txt`是自定义的停用词表,可以根据实际需要进行修改。在分词之前,先使用`set_stop_words`方法加载停用词表;在分词之后,使用`get_stop_words`方法获取停用词表,并将不在停用词表中的词加入到最终的分词结果中。
用python代码实现利用停用词词表,对分词后的文档进行去停用词。
好的,以下是一个用Python实现的基本去停用词的过程:
1. 导入必要的库:
```python
import jieba # 分词库
import os # 处理文件
```
2. 定义停用词表:
```python
stopwords_path = 'stopwords.txt' # 停用词表路径
stopwords = set()
with open(stopwords_path, encoding='utf-8') as f:
for word in f.readlines():
stopwords.add(word.strip())
```
3. 分词并去除停用词:
```python
def cut_and_remove_stopwords(filepath, stopwords):
"""
对给定的文件进行分词并去除停用词
:param filepath: 文件路径
:param stopwords: 停用词表
:return: 分词后的文本
"""
texts = ''
with open(filepath, encoding='utf-8') as f:
for line in f.readlines():
words = jieba.cut(line.strip())
for word in words:
if word not in stopwords:
texts += word + ' '
return texts
```
这个函数实现了对给定的文本文件进行分词并去除停用词的过程。它返回的是一个字符串,其中每个词之间以空格隔开。你可以根据自己的需求进行修改。
4. 使用示例:
```python
texts = cut_and_remove_stopwords('example.txt', stopwords)
```
这行代码将会对文件 "example.txt" 进行分词和去停用词处理,并将结果存储在 "texts" 变量中。
请注意,这只是一个基本实现,在实际应用中可能需要做更多的优化和调整。
阅读全文
相关推荐
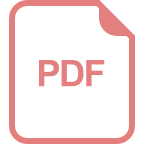












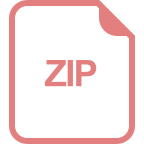
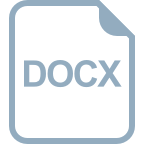