python 合并多个相同名字文件夹内容
时间: 2023-09-02 12:13:18 浏览: 293
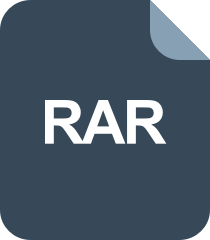
合并多个文件内容
可以使用Python的os和shutil模块来实现这个功能。具体步骤如下:
1. 获取所有需要合并的文件夹的路径。
2. 遍历每个文件夹内的所有文件,将其移动到目标文件夹内。
3. 如果出现重名文件,则可以进行文件名的重命名。
下面是一个简单的示例代码,可以实现上述功能:
```python
import os
import shutil
# 合并多个文件夹内的文件到目标文件夹内
def merge_folders(target_dir, *source_dirs):
for source_dir in source_dirs:
for root, dirs, files in os.walk(source_dir):
for file in files:
src_path = os.path.join(root, file)
dst_path = os.path.join(target_dir, file)
if os.path.exists(dst_path):
# 如果出现重名文件,则进行重命名
base, ext = os.path.splitext(dst_path)
i = 1
while os.path.exists(dst_path):
dst_path = f"{base} ({i}){ext}"
i += 1
shutil.move(src_path, dst_path)
# 测试代码
if __name__ == '__main__':
target_dir = '/path/to/your/target/directory'
source_dirs = ['/path/to/your/source/directory1', '/path/to/your/source/directory2']
merge_folders(target_dir, *source_dirs)
```
在上面的代码中,需要将`/path/to/your/target/directory`替换为你需要合并到的目标文件夹的路径,`/path/to/your/source/directory1`和`/path/to/your/source/directory2`替换为你需要合并的文件夹的路径。执行完毕后,会将所有文件夹内的文件移动到目标文件夹内,并进行重命名(如果有重名文件)。
阅读全文
相关推荐
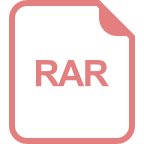

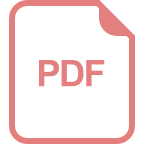
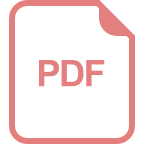
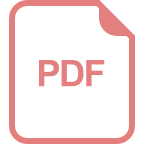









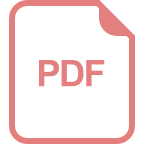
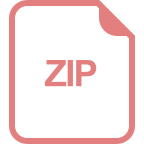
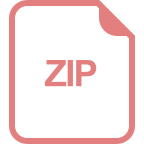
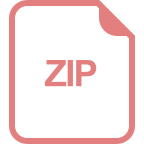