对以邻接矩阵进行 DFS 和 BFS 遍历
时间: 2023-06-15 19:08:08 浏览: 92
邻接矩阵是一种表示图的方法,其中矩阵的行和列表示图中的节点,矩阵中的值表示两个节点之间是否存在边。邻接矩阵可以用来进行深度优先搜索(DFS)和广度优先搜索(BFS)遍历。
DFS 遍历:
1. 从任意一个节点开始,标记该节点为已访问。
2. 访问该节点的所有未访问过的邻居节点,并标记这些节点为已访问。
3. 对于每个未访问过的邻居节点,重复步骤 2。
实现过程中,可以使用递归来进行 DFS 遍历。具体实现如下:
```
void dfs(int node, bool visited[], int n, int graph[][n]) {
visited[node] = true;
printf("%d ", node);
for (int i = 0; i < n; i++) {
if (graph[node][i] == 1 && !visited[i]) {
dfs(i, visited, n, graph);
}
}
}
```
其中,visited 数组用来记录每个节点是否已经被访问过,graph 是邻接矩阵。
BFS 遍历:
1. 从任意一个节点开始,将该节点加入队列中,并标记该节点为已访问。
2. 从队列中取出一个节点,访问该节点的所有未访问过的邻居节点,并将这些邻居节点加入队列中,并标记这些节点为已访问。
3. 对于每个从队列中取出的节点,重复步骤 2。
实现过程中,可以使用队列来进行 BFS 遍历。具体实现如下:
```
void bfs(int node, bool visited[], int n, int graph[][n]) {
queue<int> q;
q.push(node);
visited[node] = true;
while (!q.empty()) {
int curr = q.front();
q.pop();
printf("%d ", curr);
for (int i = 0; i < n; i++) {
if (graph[curr][i] == 1 && !visited[i]) {
visited[i] = true;
q.push(i);
}
}
}
}
```
其中,visited 数组用来记录每个节点是否已经被访问过,graph 是邻接矩阵。
阅读全文
相关推荐



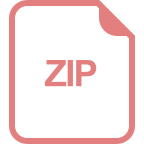
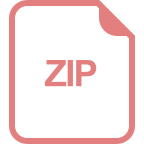
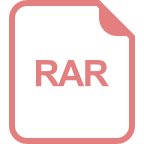
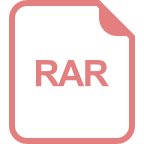
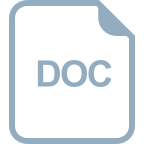
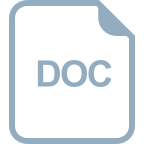


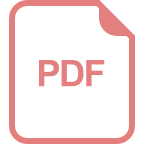
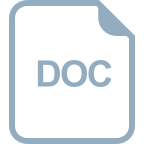


