httpclient 如何上传附件
时间: 2023-05-20 09:02:39 浏览: 53
Httpclient 可以通过 MultipartEntityBuilder 来上传附件,示例代码如下:
```
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
File file = new File(filePath);
builder.addBinaryBody("file", file, ContentType.DEFAULT_BINARY, file.getName());
HttpEntity entity = builder.build();
httpPost.setEntity(entity);
HttpResponse response = httpClient.execute(httpPost);
```
其中,url 是上传接口的地址,filePath 是要上传的文件路径。通过 addBinaryBody 方法将文件添加到请求体中,然后将请求体设置到 HttpPost 中,最后执行请求即可。
相关问题
httpclient 下载附件
您可以使用 Apache HttpClient 库来下载附件。以下是一个示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class AttachmentDownloader {
public static void main(String[] args) {
String attachmentUrl = "http://example.com/attachment.pdf";
String savePath = "path/to/save/attachment.pdf";
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpGet httpGet = new HttpGet(attachmentUrl);
HttpResponse response = httpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
if (entity != null) {
try (InputStream inputStream = entity.getContent();
OutputStream outputStream = new FileOutputStream(savePath)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
}
}
EntityUtils.consume(entity);
System.out.println("Attachment downloaded successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请确保您已经导入 Apache HttpClient 的相关依赖。在上述示例中,您需要替换 `attachmentUrl` 为要下载的附件的 URL,`savePath` 为要保存附件的本地路径。执行代码后,附件将被下载并保存在指定的路径下。
注意:在实际应用中,您可能需要添加适当的错误处理和异常处理代码,以及合适的资源释放操作来确保代码的稳健性和可靠性。
httpclient 上传文件
你可以使用 HttpClient 来上传文件。下面是一个使用 HttpClient 上传文件的示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.HttpMultipartMode;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.HttpClientBuilder;
import java.io.File;
import java.io.IOException;
public class FileUploader {
public static void main(String[] args) {
HttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost("http://example.com/upload");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
// 添加文件参数
File file = new File("path/to/file.txt");
builder.addBinaryBody("file", file, ContentType.DEFAULT_BINARY, file.getName());
HttpEntity entity = builder.build();
httpPost.setEntity(entity);
try {
HttpResponse response = httpClient.execute(httpPost);
// 处理上传结果
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
你需要将 `http://example.com/upload` 替换为你要上传文件的目标 URL,将 `"path/to/file.txt"` 替换为你要上传的文件路径。在这个示例中,我们使用了 HttpClient 4.x 版本的库进行文件上传。
相关推荐
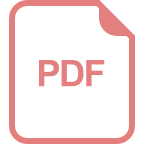
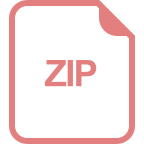












