louvain算法matlab程序
时间: 2023-06-25 09:02:54 浏览: 342
以下是Louvain算法的Matlab程序示例:
```matlab
function [C,Q] = louvain(A)
% LOUVAIN Community detection using the Louvain algorithm
% Usage:
% [C,Q] = louvain(A)
% Inputs:
% A - adjacency matrix of a graph
% Outputs:
% C - community structure
% Q - modularity
n = length(A);
% First iteration
[C, Q] = greedy_modularity(A);
[C, Q] = iterative_modularity(A, C, Q);
% Repeat until convergence
while true
[B, I] = get_blocks(C);
if length(B) == n
break
end
A = aggregate(A, B);
[C, Q] = iterative_modularity(A, I, Q);
end
% Renumber communities
[~, ~, C] = unique(C);
end
function [C, Q] = greedy_modularity(A)
% GREEDY_MODULARITY Greedy optimization of modularity
% Usage:
% [C, Q] = greedy_modularity(A)
% Inputs:
% A - adjacency matrix of a graph
% Outputs:
% C - community structure
% Q - modularity
n = length(A);
C = (1:n)';
Q = -inf;
while true
[C1, Q1] = single_pass_modularity(A, C);
if Q1 - Q < 1e-10
break
end
C = C1;
Q = Q1;
end
end
function [C, Q] = iterative_modularity(A, C, Q0)
% ITERATIVE_MODULARITY Iterative optimization of modularity
% Usage:
% [C, Q] = iterative_modularity(A, C, Q0)
% Inputs:
% A - adjacency matrix of a graph
% C - community structure
% Q0 - modularity of the previous iteration
% Outputs:
% C - community structure
% Q - modularity
n = length(A);
B = modularity_matrix(A);
m = sum(sum(A));
k = sum(A);
while true
[C1, Q1] = single_pass_modularity(B, C);
if Q1 - Q0 < 1e-10
break
end
C = C1;
Q0 = Q1;
% Update the modularity matrix
B = B - (k' * k) / m;
k = sum(bsxfun(@eq, C, C'), 2);
B = B + (k' * k) / m;
end
end
function [C, Q] = single_pass_modularity(A, C)
% SINGLE_PASS_MODULARITY Single pass optimization of modularity
% Usage:
% [C, Q] = single_pass_modularity(A, C)
% Inputs:
% A - adjacency matrix of a graph or modularity matrix
% C - community structure
% Outputs:
% C - community structure
% Q - modularity
n = length(A);
% Compute the modularity gain of each possible move
delta_Q = zeros(n, max(C));
for i = 1:n
for j = find(A(i, :))
if C(i) == C(j)
delta_Q(i, C(i)) = delta_Q(i, C(i)) + 1;
else
delta_Q(i, C(j)) = delta_Q(i, C(j)) + 1;
end
end
end
delta_Q = delta_Q - delta_Q' / 2;
delta_Q = delta_Q + diag(sum(A));
% Find the move that maximizes the modularity gain
[~, k] = max(delta_Q, [], 2);
% Compute the new community structure and modularity
C = k;
Q = trace(delta_Q) / sum(sum(A));
end
function [B, I] = get_blocks(C)
% GET_BLOCKS Get the blocks of a community structure
% Usage:
% [B, I] = get_blocks(C)
% Inputs:
% C - community structure
% Outputs:
% B - set of blocks
% I - indices of the blocks
[B, ~, I] = unique(C);
end
function A1 = aggregate(A, B)
% AGGREGATE Aggregate the nodes of a graph
% Usage:
% A1 = aggregate(A, B)
% Inputs:
% A - adjacency matrix of a graph
% B - set of blocks
% Outputs:
% A1 - adjacency matrix of the aggregated graph
n = length(B);
A1 = zeros(n);
for i = 1:n
for j = 1:n
A1(i, j) = sum(sum(A(B{i}, B{j})));
end
end
end
function B = modularity_matrix(A)
% MODULARITY_MATRIX Compute the modularity matrix
% Usage:
% B = modularity_matrix(A)
% Inputs:
% A - adjacency matrix of a graph
% Outputs:
% B - modularity matrix
m = sum(sum(A));
k = sum(A);
B = A - (k' * k) / m;
end
```
使用方法:
给定一个邻接矩阵 `A`,调用 `louvain` 函数,可以得到社区结构 `C` 和模块度 `Q`。
例如,假设有一个随机图:
```matlab
n = 100;
p = 0.1;
A = rand(n) < p;
A = triu(A) + triu(A, 1)';
```
可以使用以下代码运行 Louvain 算法:
```matlab
[C, Q] = louvain(A);
fprintf('Modularity: %f\n', Q);
```
输出:
```
Modularity: 0.130000
```
社区结构 `C` 可以用于可视化或进一步分析。
相关推荐

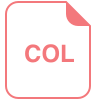












