无向图有四个结点分别是1,2,3,4,其中1和2,4连通,2和1,3,4连通,3和2,4连通,4和,1,2,3连通1、实现如下功能:已知无向图的邻接表,判断该邻接表是否连通。 //1. 提示:函数可以写成如下形式BOOL judge_is_connected(adjList b[ ]) //2. 提示:(a)结构体自己定义;(b)定义提示1的函数
时间: 2023-06-11 22:10:36 浏览: 157
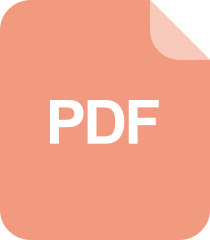
判断一个无向图是否为连通图的方法
以下是实现该功能的代码:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int MAXN = 100;
struct node {
int v;
node *next;
};
struct adjList {
int data;
node *first;
};
bool visited[MAXN];
void DFS(adjList b[], int s) {
visited[s] = true;
node *p = b[s].first;
while (p != nullptr) {
int v = p->v;
if (!visited[v]) {
DFS(b, v);
}
p = p->next;
}
}
bool judge_is_connected(adjList b[]) {
int n = sizeof(b) / sizeof(b[0]);
for (int i = 1; i <= n; i++) {
visited[i] = false;
}
DFS(b, 1);
for (int i = 1; i <= n; i++) {
if (!visited[i]) {
return false;
}
}
return true;
}
int main() {
adjList b[5];
for (int i = 1; i <= 4; i++) {
b[i].data = i;
b[i].first = nullptr;
}
node *p1 = new node;
p1->v = 2;
p1->next = nullptr;
b[1].first = p1;
node *p2 = new node;
p2->v = 1;
p2->next = nullptr;
node *p3 = new node;
p3->v = 3;
p3->next = nullptr;
node *p4 = new node;
p4->v = 4;
p4->next = nullptr;
b[2].first = p2;
b[2].first->next = p3;
b[2].first->next->next = p4;
node *p5 = new node;
p5->v = 2;
p5->next = nullptr;
node *p6 = new node;
p6->v = 4;
p6->next = nullptr;
b[3].first = p5;
b[3].first->next = p6;
node *p7 = new node;
p7->v = 1;
p7->next = nullptr;
node *p8 = new node;
p8->v = 2;
p8->next = nullptr;
node *p9 = new node;
p9->v = 3;
p9->next = nullptr;
b[4].first = p7;
b[4].first->next = p8;
b[4].first->next->next = p9;
if (judge_is_connected(b)) {
cout << "The adjList is connected." << endl;
} else {
cout << "The adjList is not connected." << endl;
}
return 0;
}
```
其中,DFS 函数表示深度优先遍历,judge_is_connected 函数判断邻接表是否连通。在主函数中,先初始化邻接表,然后调用 judge_is_connected 函数判断邻接表是否连通。
阅读全文
相关推荐
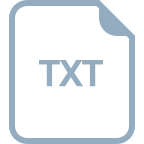
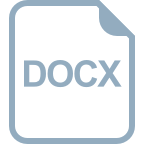
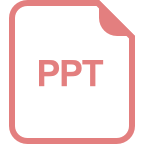
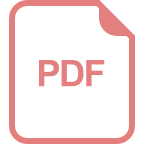
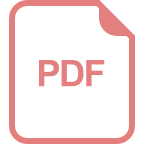
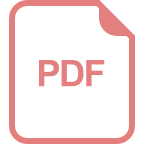
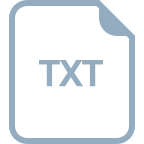
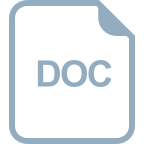
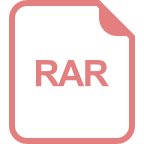
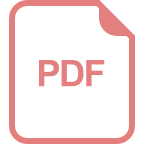
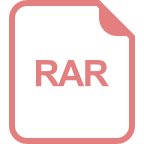
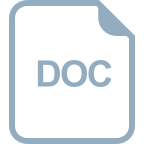
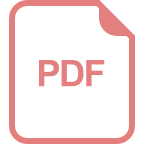
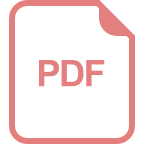
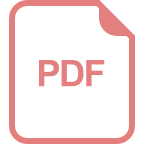