线程pthread_create()、pthread_exit()、pthread_join()、pthread_cancel()
时间: 2023-07-25 13:30:43 浏览: 67
这是关于线程的几个常用函数:
1. pthread_create():创建一个线程,参数包括线程标识符、线程属性、线程要执行的函数和函数参数。
2. pthread_exit():退出当前线程,并返回一个指向线程退出状态的指针。
3. pthread_join():等待指定线程的结束,以便获取线程的退出状态。
4. pthread_cancel():取消指定线程的执行,线程被取消后会立即停止执行。
需要注意的是,线程的创建和退出应该成对出现,不要忘记在使用 pthread_create() 创建线程之后,使用 pthread_join() 等待线程结束,并释放线程占用的资源。同时,在使用 pthread_cancel() 取消线程时,需要注意线程是否有可能在取消前就已经结束了,如果没有结束,需要在线程函数中检查取消请求并作出相应的处理。
相关问题
pthread_create,pthread_join和pthread_exit
pthread_create是一个函数,用于创建一个新的线程。它接受四个参数:指向pthread_t类型的指针thread,指向pthread_attr_t类型的指针attr,指向一个函数的指针start_routine和一个参数arg。start_routine是线程的入口函数,它接受一个void类型的指针参数并返回一个void类型的指针。pthread_create函数成功时返回0,失败时返回错误编号。
pthread_join是一个函数,用于等待一个线程的结束。它接受两个参数:pthread_t类型的参数thread和一个void类型的指针rval_ptr。rval_ptr用于获取线程的返回值。pthread_join函数会阻塞调用线程,直到指定的线程结束。成功时返回0,失败时返回错误编号。
pthread_exit是一个函数,用于终止当前线程。它接受一个void类型的指针参数rval_ptr,用于指定线程的返回值。调用pthread_exit函数会立即终止当前线程,并将指定的返回值传递给等待该线程的线程。它类似于进程的exit函数。pthread_exit函数不会返回任何值。
pthread_t *thread是一个指向pthread_t类型的指针,它用于存储线程的ID。可以使用pthread_self函数获取当前线程的ID,类似于使用getpid函数获取进程的ID。
综上所述,pthread_create用于创建新线程,pthread_join用于等待线程的结束,pthread_exit用于终止当前线程。
linux中线程pthread_exit和pthread_cancel区别
pthread_exit和pthread_cancel都可以用于终止线程,但它们之间有一些区别:
1. pthread_exit是线程主动退出,可以在线程的执行函数中调用return语句或pthread_exit函数来退出线程;而pthread_cancel是由其他线程取消目标线程的执行。
2. pthread_exit函数的作用是在线程执行结束时终止线程,而pthread_cancel函数可以在任何时候取消线程的执行。
3. pthread_exit函数的调用会使线程立即退出,而pthread_cancel函数的调用只是向目标线程发送一个取消请求,目标线程可以在取消请求到达时进行处理。
下面是一个简单的例子,演示了如何使用pthread_exit和pthread_cancel函数来终止线程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_func(void *arg)
{
int i;
for (i = 0; i < 5; i++) {
printf("Thread running %d\n", i);
sleep(1);
}
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
int ret;
ret = pthread_create(&tid, NULL, thread_func, NULL);
if (ret != 0) {
printf("Create thread error!\n");
exit(1);
}
sleep(2);
// 使用pthread_cancel函数取消线程
ret = pthread_cancel(tid);
if (ret != 0) {
printf("Cancel thread error!\n");
exit(1);
}
// 等待线程结束
ret = pthread_join(tid, NULL);
if (ret != 0) {
printf("Join thread error!\n");
exit(1);
}
printf("Thread exit!\n");
return 0;
}
```
相关推荐
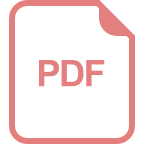
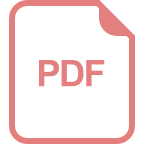
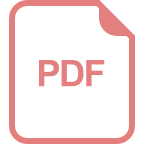












