c语言完成,直接写代码不用解释:给定一组数:{38,18,29,18,23,10,65}利用队列操作,分别实现对上述数组的数据的入队列操作,出队列操作。要求写出初始化队列的方法,入队列操作的方法,出队列操作的方法。
时间: 2023-09-24 10:06:01 浏览: 80
### 回答1:
首先,我们需要定义一个队列的结构体,这里以数组的形式实现队列:
```
#define MAX_SIZE 100
typedef struct queue {
int data[MAX_SIZE];
int head;
int tail;
} Queue;
```
然后我们需要定义初始化队列的方法:
```
void init_queue(Queue *q) {
q->head = 0;
q->tail = 0;
}
```
接下来我们定义入队列操作的方法:
```
void enqueue(Queue *q, int value) {
q->data[q->tail] = value;
q->tail++;
}
```
最后我们定义出队列操作的方法:
```
int dequeue(Queue *q) {
int ret = q->data[q->head];
q->head++;
return ret;
}
```
最终,我们可以使用如下的代码来实现对给定数组的数据的入队列操作和出队列操作:
```
int main() {
Queue q;
init_queue(&q);
int data[] = {38, 18, 29, 18, 23, 10, 65};
for (int i = 0; i < 7; i++) {
enqueue(&q, data[i]);
}
while (q.head < q.tail) {
int value = dequeue(&q);
printf("%d ", value);
}
printf("\n");
return 0;
}
```
### 回答2:
#include<stdio.h>
#include<stdlib.h>
#define MAXSIZE 100 // 定义队列的最大容量
typedef struct {
int data[MAXSIZE]; // 用数组实现队列
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = -1; // 将队头和队尾指针置为-1,表示队列为空
}
// 入队列操作
void enqueue(Queue *q, int value) {
if (q->rear == MAXSIZE - 1) {
printf("队列已满,无法入队列。\n");
return;
}
q->rear++; // 队尾指针后移
q->data[q->rear] = value; // 将元素存入队列中
}
// 出队列操作
int dequeue(Queue *q) {
if (q->front == q->rear) {
printf("队列为空,无法出队列。\n");
return -1;
}
q->front++; // 队头指针后移
return q->data[q->front]; // 返回队头元素的值
}
int main() {
int arr[] = {38, 18, 29, 18, 23, 10, 65};
int size = sizeof(arr) / sizeof(arr[0]);
Queue q;
initQueue(&q);
// 入队列操作
for (int i = 0; i < size; i++) {
enqueue(&q, arr[i]);
}
// 出队列操作
for (int i = 0; i < size; i++) {
printf("%d ", dequeue(&q));
}
return 0;
}
### 回答3:
下面是使用C语言编写的队列操作的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10
// 定义队列结构体
typedef struct {
int data[MAX_SIZE];
int front, rear;
} Queue;
// 初始化队列
void initQueue(Queue *queue) {
queue->front = -1;
queue->rear = -1;
}
// 入队列操作
void enqueue(Queue *queue, int item) {
if (queue->rear == MAX_SIZE - 1) {
printf("队列已满,无法入队列。\n");
return;
}
queue->rear++;
queue->data[queue->rear] = item;
}
// 出队列操作
int dequeue(Queue *queue) {
if (queue->front == queue->rear) {
printf("队列为空,无法出队列。\n");
return -1;
}
queue->front++;
int item = queue->data[queue->front];
return item;
}
int main() {
int nums[] = {38, 18, 29, 18, 23, 10, 65};
int size = sizeof(nums) / sizeof(nums[0]);
Queue queue;
initQueue(&queue);
// 入队列操作
for (int i = 0; i < size; i++) {
enqueue(&queue, nums[i]);
}
// 出队列操作
for (int i = 0; i < size; i++) {
int item = dequeue(&queue);
printf("%d ", item);
}
return 0;
}
```
上述代码实现了初始化队列的方法`initQueue`,入队列操作的方法`enqueue`和出队列操作的方法`dequeue`。在主函数中,首先定义了给定的一组数`nums`,然后通过循环将每个数入队列,再通过循环将每个数出队列并打印输出。
相关推荐
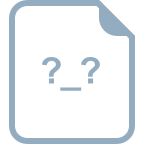














