使用c语言生成概率统计函数库
时间: 2024-02-21 10:32:05 浏览: 27
1. 随机数生成函数
```c
#include <stdlib.h>
#include <time.h>
void initRand() {
srand(time(NULL));
}
int randInt(int min, int max) {
return rand() % (max - min + 1) + min;
}
double randDouble(double min, double max) {
return (double)rand() / RAND_MAX * (max - min) + min;
}
```
2. 统计函数
```c
#include <stdio.h>
int countChar(const char* str, char c) {
int count = 0;
while (*str) {
if (*str == c) {
count++;
}
str++;
}
return count;
}
int countWord(const char* str) {
int count = 0;
while (*str) {
if (*str == ' ' || *str == '\n' || *str == '\t') {
count++;
}
str++;
}
return count;
}
int countLine(const char* str) {
int count = 0;
while (*str) {
if (*str == '\n') {
count++;
}
str++;
}
return count;
}
int countDigit(int n) {
int count = 0;
while (n) {
count++;
n /= 10;
}
return count;
}
int countPrime(int n) {
if (n <= 1) {
return 0;
}
for (int i = 2; i <= n / 2; i++) {
if (n % i == 0) {
return 0;
}
}
return 1;
}
```
3. 概率函数
```c
#include <math.h>
double normalPDF(double x, double mu, double sigma) {
return exp(-pow(x - mu, 2) / (2 * pow(sigma, 2))) / (sqrt(2 * M_PI) * sigma);
}
double normalCDF(double x, double mu, double sigma) {
return (1 + erf((x - mu) / (sigma * sqrt(2)))) / 2;
}
double binomialPDF(int k, int n, double p) {
int c = 1;
for (int i = 1; i <= k; i++) {
c *= n - i + 1;
c /= i;
}
return c * pow(p, k) * pow(1 - p, n - k);
}
double binomialCDF(int k, int n, double p) {
double cdf = 0;
for (int i = 0; i <= k; i++) {
cdf += binomialPDF(i, n, p);
}
return cdf;
}
double uniformPDF(double x, double a, double b) {
if (x < a || x > b) {
return 0;
}
return 1 / (b - a);
}
double uniformCDF(double x, double a, double b) {
if (x < a) {
return 0;
}
if (x >= b) {
return 1;
}
return (x - a) / (b - a);
}
```
4. 示例代码
```c
#include <stdio.h>
#include "stats.h"
int main() {
initRand();
// 随机数生成
printf("randInt(1, 10): %d\n", randInt(1, 10));
printf("randDouble(0, 1): %f\n", randDouble(0, 1));
// 统计函数
printf("countChar(\"hello world\", 'l'): %d\n", countChar("hello world", 'l'));
printf("countWord(\"hello world\\nhi there\\n\"): %d\n", countWord("hello world\nhi there\n"));
printf("countLine(\"hello world\\nhi there\\n\"): %d\n", countLine("hello world\nhi there\n"));
printf("countDigit(12345): %d\n", countDigit(12345));
printf("countPrime(7): %d\n", countPrime(7));
// 概率函数
printf("normalPDF(0, 0, 1): %f\n", normalPDF(0, 0, 1));
printf("normalCDF(1, 0, 1): %f\n", normalCDF(1, 0, 1));
printf("binomialPDF(2, 5, 0.5): %f\n", binomialPDF(2, 5, 0.5));
printf("binomialCDF(2, 5, 0.5): %f\n", binomialCDF(2, 5, 0.5));
printf("uniformPDF(0.5, 0, 1): %f\n", uniformPDF(0.5, 0, 1));
printf("uniformCDF(0.5, 0, 1): %f\n", uniformCDF(0.5, 0, 1));
return 0;
}
```
相关推荐
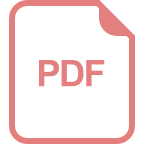















