用python写一个小恐龙代码
时间: 2024-06-05 07:12:44 浏览: 14
我可以提供一个简单的小恐龙代码:
print("欢迎来到小恐龙的世界!")
name = input("请输入你的小恐龙的名字:")
print("你的小恐龙叫作" + name + "!")
age = input("请输入你的小恐龙的年龄:")
print("你的小恐龙今年" + age + "岁!")
color = input("请输入你的小恐龙的颜色:")
print("你的小恐龙是" + color + "色的!")
相关问题
用Python写一个跳恐龙小游戏,带注释
好的,以下是使用Python写一个跳恐龙小游戏的代码,带注释说明:
```python
import pygame
import random
# 初始化game
pygame.init()
# 设置屏幕大小和标题
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Jumping Dino")
# 加载恐龙和障碍物的图像
dino_img = pygame.image.load("dino.png")
cactus_img = pygame.image.load("cactus.png")
# 设置字体和字体大小
font = pygame.font.Font(None, 40)
# 初始化游戏变量
score = 0
speed = 5
jump = False
jump_count = 10
# 定义恐龙的位置和大小
dino_x = 50
dino_y = 400
dino_width = 64
dino_height = 64
# 定义障碍物的位置和大小
cactus_x = 800
cactus_y = 410
cactus_width = 32
cactus_height = 64
# 定义函数来检测碰撞
def collision(dino_x, dino_y, cactus_x, cactus_y):
if dino_x + dino_width > cactus_x and dino_x < cactus_x + cactus_width:
if dino_y + dino_height > cactus_y:
return True
return False
# 游戏循环
running = True
while running:
# 处理退出事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 处理跳跃事件
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
jump = True
# 更新恐龙和障碍物的位置
cactus_x -= speed
if cactus_x < -cactus_width:
cactus_x = 800
score += 1
# 增加游戏难度
if score % 5 == 0:
speed += 1
# 处理跳跃
if jump:
if jump_count >= -10:
dino_y -= (jump_count * abs(jump_count)) * 0.5
jump_count -= 1
else:
jump = False
jump_count = 10
# 检测碰撞
if collision(dino_x, dino_y, cactus_x, cactus_y):
running = False
# 绘制恐龙、障碍物和分数
screen.fill((255, 255, 255))
screen.blit(dino_img, (dino_x, dino_y))
screen.blit(cactus_img, (cactus_x, cactus_y))
score_text = font.render("Score: " + str(score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 刷新屏幕
pygame.display.update()
# 退出Pygame
pygame.quit()
```
注释中已经解释了大部分代码的作用,但是需要注意一些细节:
- `pygame.init()` 用于初始化Pygame,必须放在代码的最开始。
- `pygame.display.set_mode()` 用于设置屏幕大小和标题。
- `pygame.image.load()` 用于加载图像。
- `pygame.font.Font()` 用于设置字体和字体大小。
- `pygame.event.get()` 用于获取所有的事件。
- `pygame.KEYDOWN` 用于检测按键事件。
- `screen.blit()` 用于绘制图像。
- `pygame.display.update()` 用于刷新屏幕。
此外,还需要注意跳跃的实现方式,以及障碍物的移动和生成方式。在游戏中,障碍物的移动速度和生成频率会随着游戏分数的增加而增加,这也是游戏难度的提升方式之一。
python 谷歌小恐龙代码
Python 谷歌小恐龙代码是指利用Python语言编写的仿照谷歌浏览器中断网时出现的小恐龙跳跃游戏的代码。
使用Python语言编写该小游戏的代码,通常需要使用到Pygame等相关库来实现游戏的基本功能。代码包括对游戏窗口的创建、小恐龙的绘制和控制、障碍物的生成和碰撞检测等。
首先,我们需要通过导入Pygame库来创建游戏窗口,并设置窗口的大小、背景色等基本属性。接下来,我们可以使用Pygame提供的绘图函数来绘制小恐龙和障碍物。
在游戏的循环中,需要不断监听用户的键盘输入,根据用户的操作来控制小恐龙的跳跃。当小恐龙与障碍物发生碰撞时,游戏结束,记录玩家的得分等相关信息。
此外,还需要实现对障碍物的生成和移动,以及小恐龙的重力作用和跳跃动作等。在游戏的循环中,通过不断更新小恐龙的坐标和障碍物的位置,使得游戏呈现出连续运动的效果。
最后,我们可以加入音效和计分功能,以提升游戏体验。通过不断调试和优化代码,可以使游戏具有更好的可玩性和稳定性。
总之,Python谷歌小恐龙代码是一个基于Python语言编写的仿照谷歌浏览器小恐龙跳跃游戏的代码,通过利用Pygame等库实现了游戏的基本功能,包括创建游戏窗口、绘制场景和角色、控制角色跳跃、生成障碍物、碰撞检测等。
相关推荐
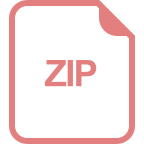
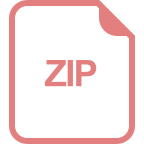












