使用drm api显示NV12数据
时间: 2023-12-11 16:03:06 浏览: 432
DRM(Direct Rendering Manager)是Linux内核中的一个子系统,它提供了一种机制,允许用户空间应用程序直接访问图形硬件。在DRM中,显示器的显示被表示为一个帧缓冲区,它是一个二维数组,其中每个元素表示一个像素的颜色值。帧缓冲区可以包含不同的像素格式,其中之一是NV12。
要在DRM中显示NV12数据,您需要使用DRM API的drmModeAddFB2函数创建一个新的帧缓冲区对象,并将NV12数据附加到该对象。以下是一个简单的示例程序,演示如何使用DRM API显示NV12数据:
```c
#include <fcntl.h>
#include <stdint.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <drm/drm_fourcc.h>
#include <drm/drm_mode.h>
#define WIDTH 640
#define HEIGHT 480
int main(int argc, char **argv)
{
int fd, ret;
fd = open("/dev/dri/card0", O_RDWR);
if (fd < 0) {
fprintf(stderr, "failed to open DRM device\n");
return 1;
}
uint32_t handles[4] = { 0 };
uint32_t pitches[4] = { 0 };
uint32_t offsets[4] = { 0 };
uint64_t modifier = DRM_FORMAT_MOD_NONE;
struct drm_mode_create_dumb create = {
.width = WIDTH,
.height = HEIGHT,
.bpp = 16,
};
struct drm_mode_map_dumb map = { 0 };
struct drm_mode_fb_cmd2 cmd = { 0 };
uint8_t *src, *dst;
ret = ioctl(fd, DRM_IOCTL_MODE_CREATE_DUMB, &create);
if (ret < 0) {
fprintf(stderr, "failed to create dumb buffer: %m\n");
goto out_close;
}
cmd.width = WIDTH;
cmd.height = HEIGHT;
cmd.pixel_format = DRM_FORMAT_NV12;
cmd.flags = DRM_MODE_FB_MODIFIERS;
cmd.handles[0] = create.handle;
cmd.pitches[0] = create.pitch;
cmd.modifier[0] = modifier;
ret = ioctl(fd, DRM_IOCTL_MODE_ADDFB2, &cmd);
if (ret < 0) {
fprintf(stderr, "failed to add framebuffer: %m\n");
goto out_destroy;
}
map.handle = create.handle;
ret = ioctl(fd, DRM_IOCTL_MODE_MAP_DUMB, &map);
if (ret < 0) {
fprintf(stderr, "failed to map dumb buffer: %m\n");
goto out_rmfb;
}
src = malloc(WIDTH * HEIGHT * 3 / 2);
dst = mmap(NULL, create.size, PROT_WRITE, MAP_SHARED, fd, map.offset);
if (dst == MAP_FAILED) {
fprintf(stderr, "failed to mmap framebuffer: %m\n");
goto out_unmap;
}
/* fill NV12 buffer with some data */
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
src[y * WIDTH + x] = x + y;
}
}
for (int y = 0; y < HEIGHT / 2; y++) {
for (int x = 0; x < WIDTH; x += 2) {
src[WIDTH * HEIGHT + y * WIDTH + x] = y;
src[WIDTH * HEIGHT + y * WIDTH + x + 1] = y + 128;
}
}
/* copy NV12 buffer to framebuffer */
for (int y = 0; y < HEIGHT; y++) {
memcpy(dst + y * create.pitch, src + y * WIDTH, WIDTH);
}
for (int y = 0; y < HEIGHT / 2; y++) {
memcpy(dst + create.pitch * (HEIGHT + y), src + WIDTH * HEIGHT + y * WIDTH, WIDTH);
}
munmap(dst, create.size);
free(src);
close(fd);
return 0;
out_unmap:
munmap(dst, create.size);
out_rmfb:
ioctl(fd, DRM_IOCTL_MODE_RMFB, &cmd.fb_id);
out_destroy:
ioctl(fd, DRM_IOCTL_MODE_DESTROY_DUMB, &create);
out_close:
close(fd);
return 1;
}
```
此程序使用DRM API创建一个NV12格式的帧缓冲区对象,并将其映射到用户空间。然后,它填充一个NV12格式的缓冲区,将其复制到帧缓冲区中,并清理所有资源。注意,此程序仅用于演示目的,不应在生产环境中使用。
阅读全文
相关推荐
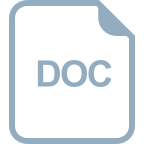
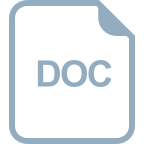
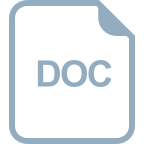
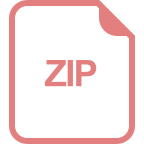
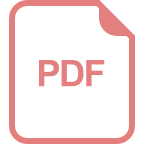
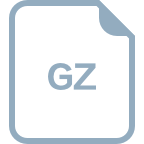
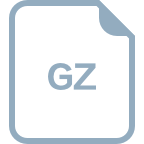
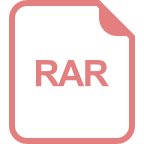
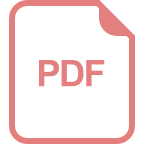
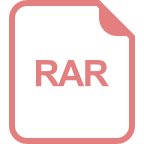
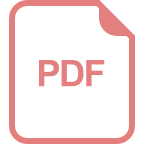




