对npz文件通过python读取prediction和truth并输出
时间: 2023-07-18 09:02:56 浏览: 145
假设npz文件中有以下两个键值对:`prediction`和`truth`。
可以使用以下代码来读取这些值并输出:
```python
import numpy as np
# 加载npz文件
data = np.load('example.npz')
# 读取预测值
prediction = data['prediction']
print('Prediction:', prediction)
# 读取真实值
truth = data['truth']
print('Truth:', truth)
```
请注意,如果npz文件中有多个键值对,则需要使用`data.keys()`来查看所有的键,并使用相应的键来访问对应的值。
相关问题
npz文件中数组为Prediction shape: (3408, 12, 307, 1) Truth shape: (3408, 12, 307, 1),读取并输出为图像
可以使用numpy和PIL库来读取并输出为图像。
首先,我们需要加载npz文件中的数组:
```python
import numpy as np
data = np.load('filename.npz')
prediction = data['Prediction']
truth = data['Truth']
```
接下来,我们将数组转换为图像。假设每个数组元素的取值范围是0到255之间的整数,我们可以使用PIL库将它们转换为图像:
```python
from PIL import Image
def array_to_image(arr):
# 将数组元素的取值范围从[0, 255]映射到[0, 1]
arr = arr.astype(np.float32) / 255.0
# 创建PIL图像对象
img = Image.fromarray(np.uint8(arr * 255.0))
return img
```
现在,我们可以将两个数组中的每个元素转换为图像并保存:
```python
import os
# 创建保存图像的目录
if not os.path.exists('images'):
os.makedirs('images')
# 遍历数组中的每个元素并保存为图像
for i in range(prediction.shape[0]):
for j in range(prediction.shape[1]):
# 将预测结果和真实值分别转换为图像
pred_img = array_to_image(prediction[i, j])
truth_img = array_to_image(truth[i, j])
# 保存图像
pred_img.save(f'images/prediction_{i}_{j}.png')
truth_img.save(f'images/truth_{i}_{j}.png')
```
这样,我们就将npz文件中的数组转换为图像并保存到了本地。
npz文件中数组为Prediction shape: (3408, 12, 307, 1) Truth shape: (3408, 12, 307, 1),读取并合并两个数组输出为折线图并给出完整代码
首先,我们需要使用numpy和matplotlib库来读取npz文件和绘制折线图。以下是完整代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取npz文件
data = np.load('data.npz')
prediction = data['Prediction']
truth = data['Truth']
# 合并数组
merged = np.concatenate((prediction, truth), axis=3)
# 绘制折线图
x = np.arange(0, 307)
for i in range(12):
plt.plot(x, merged[0, i, :, 0], label='Prediction' if i == 0 else None)
plt.plot(x, merged[0, i, :, 1], label='Truth' if i == 0 else None)
plt.legend()
plt.show()
```
解释一下代码:
首先,我们使用numpy库的`load`函数来读取npz文件,并将其存储在变量`data`中。我们可以通过使用`data['Prediction']`和`data['Truth']`来获取文件中的两个数组。
接下来,我们使用numpy库的`concatenate`函数来将两个数组合并成一个新的数组。由于我们想要在最后一个维度上合并两个数组,我们将`axis`参数设置为3。
最后,我们使用matplotlib库来绘制折线图。对于每个时间步,我们使用`plt.plot`函数来绘制两条曲线,一条代表预测值,一条代表真实值。我们还使用`label`参数来为每条曲线添加标签。最后,我们使用`plt.legend()`函数来添加图例,并使用`plt.show()`函数来显示图形。
阅读全文
相关推荐
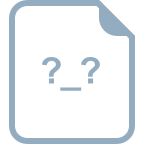
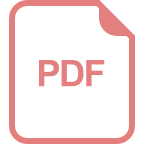




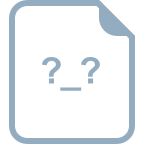
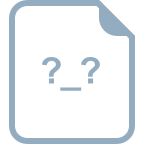
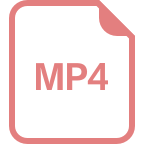