stm32串口1接收命令然后发送从串口2接收到的数据
时间: 2023-11-12 16:09:15 浏览: 118
实现这个功能的方法,可以分为以下几个步骤:
1. 配置串口1和串口2的硬件参数,包括波特率、数据位、停止位、奇偶校验等。
2. 在代码中开启串口1和串口2的中断接收功能,并编写中断服务函数来处理接收到的数据。
3. 在串口1的中断服务函数中,判断接收到的数据是否为命令,如果是,则执行相应的操作。
4. 在串口2的中断服务函数中,将接收到的数据存储到缓冲区中。
5. 在串口1的命令处理函数中,向串口2发送数据,并将串口2接收到的数据发送回串口1。
下面是一份示例代码,可以供您参考:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define USART1_RX_BUF_SIZE 64
#define USART2_RX_BUF_SIZE 64
uint8_t usart1_rx_buf[USART1_RX_BUF_SIZE];
uint8_t usart2_rx_buf[USART2_RX_BUF_SIZE];
volatile uint8_t usart1_rx_len = 0;
volatile uint8_t usart2_rx_len = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
uint8_t data = USART_ReceiveData(USART1);
if (usart1_rx_len == 0 && data != 0x55)
{
return;
}
usart1_rx_buf[usart1_rx_len++] = data;
if (usart1_rx_len == 4)
{
if (usart1_rx_buf[0] == 0x55 && usart1_rx_buf[1] == 0xAA)
{
if (usart1_rx_buf[2] == 0x01 && usart1_rx_buf[3] == 0x00)
{
// 接收到命令,执行相应的操作
USART_SendData(USART2, 0x01);
}
usart1_rx_len = 0;
}
else
{
usart1_rx_len = 0;
}
}
}
}
void USART2_IRQHandler(void)
{
if (USART_GetITStatus(USART2, USART_IT_RXNE) != RESET)
{
uint8_t data = USART_ReceiveData(USART2);
if (usart2_rx_len < USART2_RX_BUF_SIZE)
{
usart2_rx_buf[usart2_rx_len++] = data;
}
}
}
void USART1_Init(void)
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
void USART2_Init(void)
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE);
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
int main(void)
{
USART1_Init();
USART2_Init();
while (1)
{
if (usart2_rx_len > 0)
{
USART_SendData(USART1, usart2_rx_buf[usart2_rx_len - 1]);
usart2_rx_len--;
}
}
}
```
在这份代码中,我们通过开启USART1和USART2的中断接收功能,并编写中断服务函数来处理接收到的数据。当接收到的数据符合特定的格式时,我们就执行相应的操作,并向USART2发送数据。在主函数中,我们不断地从USART2接收数据,并将其发送回USART1。
阅读全文
相关推荐
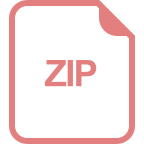
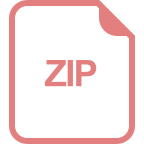
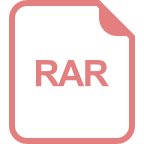














