with torch.no_grad(): for inputs, labels in test_loader: outputs = model(inputs) _, predicted = torch.max(outputs.data, 1) test_true.extend(labels.numpy()) test_pred.extend(predicted.numpy())
时间: 2023-06-07 18:11:19 浏览: 148
您的问题是一个代码段,它是用 Python 编写的,用于测试机器学习模型的精度。在这个代码段中,使用了 PyTorch 框架来加载测试数据并进行模型预测。使用 "torch.no_grad()" 函数可以避免模型对测试数据集的梯度进行更新。测试数据集包括输入和标签,模型对输入进行预测,并与真实标签进行比较,以计算模型的精度。最终,预测结果被存储在 test_pred 列表中,真实标签被存储在 test_true 列表中。
相关问题
with torch.no_grad():
The `torch.no_grad()` context manager is used to turn off gradient computation during model inference, i.e., when we are evaluating a model on test data or making predictions. When inside the `torch.no_grad()` context, any operation that requires gradient computation will not be tracked by PyTorch's autograd engine. This can help reduce memory usage and speed up computation, as we don't need to store intermediate values for backpropagation.
Here's an example of how we might use `torch.no_grad()` during model inference:
```
model.eval() # Set the model to evaluation mode
with torch.no_grad():
for inputs, labels in test_loader:
# Perform forward pass to get predictions
outputs = model(inputs)
# Compute test set accuracy
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Test Accuracy: {:.2f}%'.format(100 * correct / total))
```
In this example, we use `torch.no_grad()` to compute the test set accuracy without tracking the gradients of the model's parameters. This can help speed up the computation and reduce memory usage, especially if we have a large test set.
解释下面的代码correct = 0 total = 0 with torch.no_grad(): for inputs, labels in test_loader: inputs, labels = inputs.float(), labels.long() outputs = net(inputs) _, predicted = torch.max(outputs.data, 1) total += labels.size(0) correct += (predicted == labels).sum().item() print('Accuracy of the network: %d %%' % ( 100 * correct / total))
这段代码是用于计算神经网络在测试集上的分类准确率的。
首先,定义了两个变量correct和total,用于记录测试集上分类正确的样本数和总样本数。
然后,使用了with torch.no_grad()来关闭梯度计算,以便加快代码运行速度和减少内存占用。
接着,使用for循环遍历测试集中的每个样本,将输入数据inputs和标签labels传入神经网络net中进行前向传播,并获取输出值outputs。利用torch.max()函数获取输出值outputs每行中最大值和对应的索引,即预测的标签predicted。
再接下来,累加变量total的值,以记录测试集中样本的总数。同时,使用(predicted == labels).sum().item()来统计预测正确的样本数,将其累加到变量correct中。
最后,计算神经网络在测试集上的分类准确率,并打印输出。
需要注意的是,在代码中将inputs转化为float类型,labels转化为long类型,以与神经网络的输入和输出类型相匹配。同时,使用predicted == labels来比较预测值和真实标签是否相同,统计预测正确的样本数。最后计算准确率时,将correct除以total并乘以100,输出百分数形式的准确率。
阅读全文
相关推荐
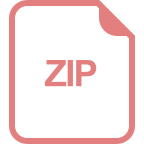
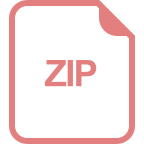
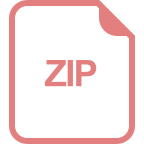













