netty自定义协议demo
时间: 2023-09-15 07:20:14 浏览: 176
以下是一个简单的Netty自定义协议的示例代码,该协议由消息头和消息体组成,消息头包含消息的长度信息,消息体包含具体的消息内容。
1. 定义消息类
```java
public class MyMessage {
private int length;
private String content;
// getter and setter methods
}
```
2. 编写编解码器
```java
public class MyMessageCodec extends MessageToByteEncoder<MyMessage> {
@Override
protected void encode(ChannelHandlerContext ctx, MyMessage msg, ByteBuf out) throws Exception {
byte[] contentBytes = msg.getContent().getBytes(CharsetUtil.UTF_8);
out.writeInt(contentBytes.length);
out.writeBytes(contentBytes);
}
}
public class MyMessageDecoder extends ByteToMessageDecoder {
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List<Object> out) throws Exception {
if (in.readableBytes() < 4) {
return;
}
in.markReaderIndex();
int length = in.readInt();
if (in.readableBytes() < length) {
in.resetReaderIndex();
return;
}
byte[] contentBytes = new byte[length];
in.readBytes(contentBytes);
String content = new String(contentBytes, CharsetUtil.UTF_8);
MyMessage message = new MyMessage();
message.setLength(length);
message.setContent(content);
out.add(message);
}
}
```
3. 编写服务端和客户端代码
服务端代码:
```java
public class MyServer {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new MyMessageDecoder());
pipeline.addLast(new MyServerHandler());
pipeline.addLast(new MyMessageCodec());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(8888).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
public class MyServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
MyMessage message = (MyMessage) msg;
System.out.println("Server receive message: " + message.getContent());
message.setContent("Hello, " + message.getContent() + "!");
ctx.writeAndFlush(message);
}
}
```
客户端代码:
```java
public class MyClient {
public static void main(String[] args) throws Exception {
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(workerGroup)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new MyMessageCodec());
pipeline.addLast(new MyMessageDecoder());
pipeline.addLast(new MyClientHandler());
}
});
ChannelFuture f = b.connect("localhost", 8888).sync();
for (int i = 0; i < 10; i++) {
MyMessage message = new MyMessage();
message.setContent("world-" + i);
f.channel().writeAndFlush(message);
Thread.sleep(1000);
}
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
}
}
}
public class MyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
MyMessage message = (MyMessage) msg;
System.out.println("Client receive message: " + message.getContent());
}
}
```
以上代码演示了如何使用Netty实现自定义协议,其中MyMessageCodec和MyMessageDecoder负责编解码,MyServer和MyServerHandler负责服务端逻辑,MyClient和MyClientHandler负责客户端逻辑。在本示例中,自定义协议包含消息头和消息体,消息头包含消息长度信息,消息体包含具体的消息内容。
阅读全文
相关推荐
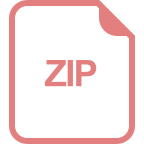
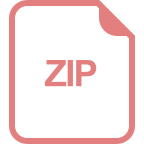
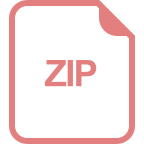
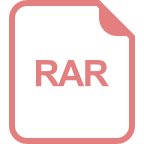
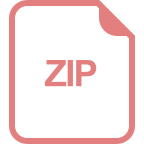
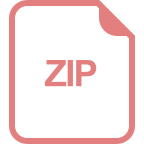
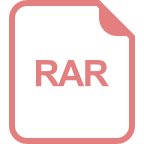
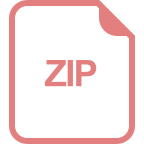
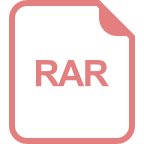
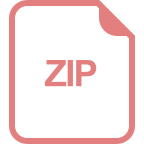
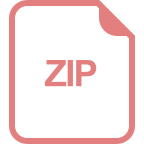
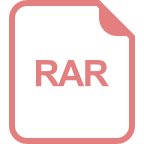
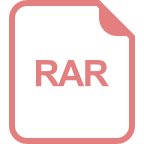
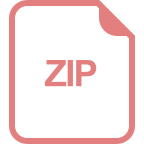
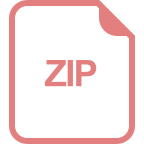
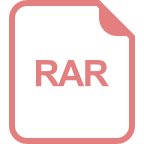
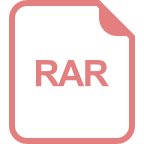