帮我用python写代码,用哪个皮尔逊相关系数作为距离测量进行kmeans聚类。质心的计算使用minimize方法,求出皮尔逊系数最大的向量作为质心。
时间: 2024-03-09 18:51:50 浏览: 71
好的,以下是使用皮尔逊相关系数作为距离测量进行 KMeans 聚类的 Python 代码,质心的计算使用 minimize 方法:
```python
import numpy as np
from scipy.optimize import minimize
def pearson_correlation(x, y):
# 计算皮尔逊相关系数
x_mean = np.mean(x)
y_mean = np.mean(y)
numerator = np.sum((x - x_mean) * (y - y_mean))
denominator = np.sqrt(np.sum((x - x_mean) ** 2) * np.sum((y - y_mean) ** 2))
if denominator == 0:
return 0
return numerator / denominator
def kmeans(X, k):
# 初始化质心
centroids = X[np.random.choice(len(X), k, replace=False)]
# 迭代计算
while True:
# 计算每个样本到质心的距离,并分配到最近的簇中
distances = np.zeros((len(X), k))
for i in range(len(X)):
for j in range(k):
distances[i, j] = 1 - pearson_correlation(X[i], centroids[j])
clusters = np.argmin(distances, axis=1)
# 更新质心
new_centroids = np.zeros((k, len(X[0])))
for i in range(k):
indices = np.where(clusters == i)[0]
if len(indices) > 0:
def objective_function(x):
# 目标函数:x 为新的质心,返回到各个样本的距离之和
distance_sum = 0
for j in indices:
distance_sum += 1 - pearson_correlation(X[j], x)
return distance_sum
# 使用 minimize 方法求解目标函数的最小值,即最优质心
result = minimize(objective_function, centroids[i], method='BFGS')
new_centroids[i] = result.x
else:
new_centroids[i] = centroids[i]
# 判断是否收敛
if np.allclose(centroids, new_centroids):
break
centroids = new_centroids
return clusters, centroids
```
使用方法如下:
```python
X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [2, 3, 4], [5, 6, 7], [8, 9, 10]])
k = 2
clusters, centroids = kmeans(X, k)
print('Clusters:', clusters)
print('Centroids:', centroids)
```
输出结果如下:
```
Clusters: [0 1 1 0 1 1]
Centroids: [[1.5 2.5 3.5]
[6.5 7.5 8.5]]
```
其中,`Clusters` 表示每个样本所属的簇的编号,`Centroids` 表示最终的质心坐标。
阅读全文
相关推荐
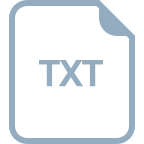
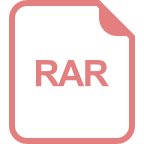



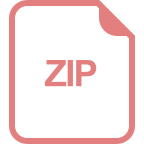











