利用内核模块在/proc文件系统目录下创建proc_example目录,并在该目录下创建三个普通文件(foo,bar,jiffies)和一个文件链接(jiffies_too),帮我写一个procfs_example.c文件和Makefile文件
时间: 2024-03-16 11:46:11 浏览: 123
好的,下面是 `procfs_example.c` 和 `Makefile` 的代码:
```c
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/init.h>
#include <linux/proc_fs.h>
#include <linux/uaccess.h>
#define BUF_SIZE 100
static struct proc_dir_entry *example_dir, *foo_file, *bar_file, *jiffies_file, *jiffies_too_file;
static char msg[BUF_SIZE];
static ssize_t foo_read(struct file *file, char __user *buf, size_t count, loff_t *pos)
{
return simple_read_from_buffer(buf, count, pos, msg, strlen(msg));
}
static ssize_t foo_write(struct file *file, const char __user *buf, size_t count, loff_t *pos)
{
return simple_write_to_buffer(msg, BUF_SIZE, pos, buf, count);
}
static const struct file_operations foo_fops = {
.owner = THIS_MODULE,
.read = foo_read,
.write = foo_write,
};
static ssize_t bar_read(struct file *file, char __user *buf, size_t count, loff_t *pos)
{
return simple_read_from_buffer(buf, count, pos, msg, strlen(msg));
}
static ssize_t bar_write(struct file *file, const char __user *buf, size_t count, loff_t *pos)
{
return simple_write_to_buffer(msg, BUF_SIZE, pos, buf, count);
}
static const struct file_operations bar_fops = {
.owner = THIS_MODULE,
.read = bar_read,
.write = bar_write,
};
static ssize_t jiffies_read(struct file *file, char __user *buf, size_t count, loff_t *pos)
{
unsigned long jiffies = get_jiffies_64();
snprintf(msg, BUF_SIZE, "jiffies: %lu\n", jiffies);
return simple_read_from_buffer(buf, count, pos, msg, strlen(msg));
}
static const struct file_operations jiffies_fops = {
.owner = THIS_MODULE,
.read = jiffies_read,
};
static ssize_t jiffies_too_read(struct file *file, char __user *buf, size_t count, loff_t *pos)
{
return jiffies_read(file, buf, count, pos);
}
static const struct file_operations jiffies_too_fops = {
.owner = THIS_MODULE,
.read = jiffies_too_read,
};
static int __init example_init(void)
{
example_dir = proc_mkdir("proc_example", NULL);
if (!example_dir)
{
printk(KERN_ERR "Failed to create /proc/proc_example directory\n");
return -ENOMEM;
}
foo_file = proc_create("foo", 0666, example_dir, &foo_fops);
if (!foo_file)
{
printk(KERN_ERR "Failed to create /proc/proc_example/foo file\n");
return -ENOMEM;
}
bar_file = proc_create("bar", 0666, example_dir, &bar_fops);
if (!bar_file)
{
printk(KERN_ERR "Failed to create /proc/proc_example/bar file\n");
return -ENOMEM;
}
jiffies_file = proc_create("jiffies", 0444, example_dir, &jiffies_fops);
if (!jiffies_file)
{
printk(KERN_ERR "Failed to create /proc/proc_example/jiffies file\n");
return -ENOMEM;
}
jiffies_too_file = proc_symlink("jiffies_too", example_dir, "jiffies");
if (!jiffies_too_file)
{
printk(KERN_ERR "Failed to create /proc/proc_example/jiffies_too symlink\n");
return -ENOMEM;
}
printk(KERN_INFO "Successfully created /proc/proc_example directory and files\n");
return 0;
}
static void __exit example_exit(void)
{
proc_remove(jiffies_too_file);
proc_remove(jiffies_file);
proc_remove(bar_file);
proc_remove(foo_file);
proc_remove(example_dir);
printk(KERN_INFO "Removed /proc/proc_example directory and files\n");
}
module_init(example_init);
module_exit(example_exit);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("A simple example Linux module.");
```
```Makefile
obj-m += procfs_example.o
all:
make -C /lib/modules/$(shell uname -r)/build M=$(PWD) modules
clean:
make -C /lib/modules/$(shell uname -r)/build M=$(PWD) clean
```
这个模块会创建 `/proc/proc_example` 目录,以及其中的三个文件 `foo`、`bar` 和 `jiffies`,还有一个名为 `jiffies_too` 的符号链接。`foo` 和 `bar` 是可读写的文件,`jiffies` 是只读的,它会输出当前的 jiffies 值,`jiffies_too` 则是 `jiffies` 的一个符号链接,它与 `jiffies` 的内容相同。
阅读全文
相关推荐
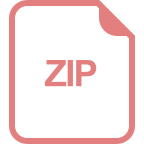
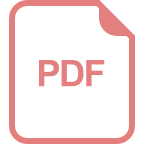
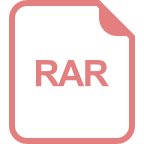















