帮我写一个Java烟花代码
时间: 2023-03-27 22:03:24 浏览: 111
好的,以下是一个简单的Java烟花代码:
```
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Firework extends JPanel implements Runnable {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int MAX_PARTICLES = 200;
private static final int GRAVITY = 1;
private static final int MAX_VELOCITY = 10;
private static final int MAX_EXPLOSION_RADIUS = 100;
private static final int MIN_EXPLOSION_RADIUS = 20;
private static final int MAX_EXPLOSION_PARTICLES = 50;
private static final int MIN_EXPLOSION_PARTICLES = 10;
private static final int MAX_FIREWORKS = 10;
private static final int MIN_FIREWORKS = 5;
private static final int FIREWORK_DELAY = 100;
private static final Color[] COLORS = { Color.RED, Color.ORANGE, Color.YELLOW, Color.GREEN, Color.BLUE, Color.MAGENTA };
private ArrayList<Particle> particles;
private Point position;
private int velocity;
private int explosionRadius;
private int explosionParticles;
private Color color;
private boolean exploded;
private int fireworksCount;
private Random random;
public Firework() {
particles = new ArrayList<Particle>();
random = new Random();
position = new Point(random.nextInt(WIDTH), HEIGHT);
velocity = random.nextInt(MAX_VELOCITY) + 1;
explosionRadius = random.nextInt(MAX_EXPLOSION_RADIUS - MIN_EXPLOSION_RADIUS) + MIN_EXPLOSION_RADIUS;
explosionParticles = random.nextInt(MAX_EXPLOSION_PARTICLES - MIN_EXPLOSION_PARTICLES) + MIN_EXPLOSION_PARTICLES;
color = COLORS[random.nextInt(COLORS.length)];
exploded = false;
fireworksCount = random.nextInt(MAX_FIREWORKS - MIN_FIREWORKS) + MIN_FIREWORKS;
}
public void run() {
try {
Thread.sleep(FIREWORK_DELAY);
} catch (InterruptedException e) {
e.printStackTrace();
}
while (fireworksCount > ) {
if (!exploded) {
position.y -= velocity;
velocity -= GRAVITY;
if (velocity <= ) {
exploded = true;
explode();
}
} else {
for (int i = ; i < particles.size(); i++) {
Particle p = particles.get(i);
p.update();
if (p.getRadius() <= ) {
particles.remove(i);
}
}
if (particles.size() == ) {
fireworksCount--;
reset();
}
}
repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void explode() {
for (int i = ; i < explosionParticles; i++) {
particles.add(new Particle(position.x, position.y, explosionRadius, color));
}
}
private void reset() {
particles.clear();
position = new Point(random.nextInt(WIDTH), HEIGHT);
velocity = random.nextInt(MAX_VELOCITY) + 1;
explosionRadius = random.nextInt(MAX_EXPLOSION_RADIUS - MIN_EXPLOSION_RADIUS) + MIN_EXPLOSION_RADIUS;
explosionParticles = random.nextInt(MAX_EXPLOSION_PARTICLES - MIN_EXPLOSION_PARTICLES) + MIN_EXPLOSION_PARTICLES;
color = COLORS[random.nextInt(COLORS.length)];
exploded = false;
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (int i = ; i < particles.size(); i++) {
Particle p = particles.get(i);
g.setColor(p.getColor());
g.fillOval(p.getX() - p.getRadius(), p.getY() - p.getRadius(), p.getRadius() * 2, p.getRadius() * 2);
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Fireworks");
frame.setSize(WIDTH, HEIGHT);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Firework fireworks = new Firework();
frame.add(fireworks);
frame.setVisible(true);
new Thread(fireworks).start();
}
}
class Particle {
private int x;
private int y;
private int radius;
private Color color;
private int velocityX;
private int velocityY;
public Particle(int x, int y, int radius, Color color) {
this.x = x;
this.y = y;
this.radius = radius;
this.color = color;
velocityX = (int) (Math.random() * 20) - 10;
velocityY = (int) (Math.random() * 20) - 10;
}
public void update() {
x += velocityX;
y += velocityY;
radius--;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getRadius() {
return radius;
}
public Color getColor() {
return color;
}
}
```
阅读全文
相关推荐
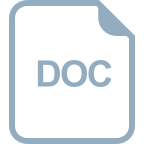
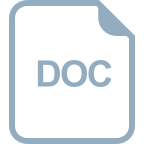
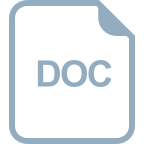













