编写int getMax(int score[])方法,用于返回score数组的最大值。在main方法中用数组stuScore[]进行测试,stuScore中的元素为:59、66、73、84、95;
时间: 2024-02-16 14:03:05 浏览: 74
好的,下面是编写的代码:
```c++
#include <iostream>
using namespace std;
int getMax(int score[]) {
int maxScore = score[0];
for (int i = 1; i < 5; i++) {
if (score[i] > maxScore) {
maxScore = score[i];
}
}
return maxScore;
}
int main() {
int stuScore[] = {59, 66, 73, 84, 95};
int maxScore = getMax(stuScore);
cout << "最高分是:" << maxScore << endl;
return 0;
}
```
该代码定义了一个名为 `getMax` 的函数,参数为一个整型数组 `score`。函数通过遍历数组,找到数组中的最大值并返回。在 `main` 函数中,我们定义了一个名为 `stuScore` 的整型数组,包含了5个分数。接着调用 `getMax` 函数得到最大值,并输出到屏幕上。
需要注意的是,此处我们假设数组中的元素数量为5,如果数组中元素数量不固定,需要修改循环的终止条件,例如可以采用 `sizeof(score) / sizeof(score[0])` 来获取数组长度。
相关问题
java编写int getMax(int score[])方法,用于返回score数组的最大值。在main方法中用数组stuScore[]进行测试,stuScore中的元素为:59、66、73、84、95;
好的,下面是Java语言的代码实现:
```java
public class Main {
public static int getMax(int[] score) {
int maxScore = score[0];
for (int i = 1; i < score.length; i++) {
if (score[i] > maxScore) {
maxScore = score[i];
}
}
return maxScore;
}
public static void main(String[] args) {
int[] stuScore = {59, 66, 73, 84, 95};
int maxScore = getMax(stuScore);
System.out.println("最高分是:" + maxScore);
}
}
```
该代码定义了一个名为 `getMax` 的静态方法,参数为一个整型数组 `score`。函数通过遍历数组,找到数组中的最大值并返回。在 `main` 函数中,我们定义了一个名为 `stuScore` 的整型数组,包含了5个分数。接着调用 `getMax` 方法得到最大值,并输出到屏幕上。
需要注意的是,在Java中,数组长度可以通过 `score.length` 获取,因此我们可以直接使用该属性作为循环的终止条件,无需手动指定数组长度。
在getmax.c中编写函数int getmax(int a[],int n),获取数组a的最大值
在`getmax.c`中,如果你想要编写一个函数`int getmax(int a[], int n)`,目的是找到数组`a`中的最大值,你可以这样做:
首先,你需要对整个数组进行遍历,依次比较每个元素,将其与当前已知的最大值进行比较。当遇到比当前最大值更大的元素时,就更新最大值。以下是一个简单的示例:
```c
#include <stdio.h>
#include <assert.h> // 添加此行用于防止数组越界错误
int get_max(int a[], int n) {
if (n <= 0) { // 验证输入数组长度是否有效
printf("Error: Array size should be positive.\n");
return -1; // 或者抛出异常,具体看项目需求
}
int max_value = a[0]; // 初始假设第一个元素是最大的
for (int i = 1; i < n; i++) {
if (a[i] > max_value) {
max_value = a[i];
}
}
return max_value;
}
int main() {
int array[] = {5, 10, 1, 7, 3};
int n = sizeof(array) / sizeof(array[0]);
int max_in_array = get_max(array, n);
if (max_in_array != -1) {
printf("The maximum value in the array is: %d\n", max_in_array);
}
return 0;
}
```
在这个示例里,`get_max`函数会返回数组`a`中的最大值。注意,为了处理数组越界的情况,我们添加了一个检查,当`n`为0或负数时,函数会返回错误信息或者特殊值表示无效。
阅读全文
相关推荐
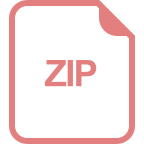













