在动态整型数组类 CArray 中,添加深拷贝构造函数 设计一个动态整型数组类 CArray ,并对CArray类进行测试 数据成员: int *vec; // 动态数组指针 int size; //动态数组规模 成员函数:public CArray(int n); // 构造函数,构造规模为n的数组 CArray (CArray &arr); //拷贝构造函数 ~CArray(); //析构函数,释放动态数组空间 int length(); // 取数组元素个数 int get(int i); // 取数组第i个元素 void set(int i, int value); // 设置数组第i个元素 int getMax(); // 取数组最大元素 int getMin(); // 取数组最大元素 void print(); //输出动态数组的所有元素 int & operator[](int); CArray & operator=( const CArray &arr);
时间: 2023-06-19 16:07:24 浏览: 166
下面是实现了深拷贝构造函数的 CArray 类:
```cpp
#include <iostream>
#include <algorithm> // for std::max and std::min
class CArray {
public:
// Constructor
CArray(int n) : size(n) {
vec = new int[size];
}
// Copy constructor (deep copy)
CArray(const CArray &arr) : size(arr.size) {
vec = new int[size];
std::copy(arr.vec, arr.vec + size, vec);
}
// Destructor
~CArray() {
delete[] vec;
}
// Get the number of elements in the array
int length() const {
return size;
}
// Get the i-th element of the array
int get(int i) const {
return vec[i];
}
// Set the i-th element of the array to value
void set(int i, int value) {
vec[i] = value;
}
// Get the maximum element in the array
int getMax() const {
return *std::max_element(vec, vec + size);
}
// Get the minimum element in the array
int getMin() const {
return *std::min_element(vec, vec + size);
}
// Print all elements in the array
void print() const {
for (int i = 0; i < size; ++i) {
std::cout << vec[i] << " ";
}
std::cout << std::endl;
}
// Overload the subscript operator [] to access elements in the array
int & operator[](int i) {
return vec[i];
}
// Overload the assignment operator = to perform deep copy
CArray & operator=(const CArray &arr) {
if (this != &arr) {
delete[] vec;
size = arr.size;
vec = new int[size];
std::copy(arr.vec, arr.vec + size, vec);
}
return *this;
}
private:
int *vec; // Dynamic array pointer
int size; // Size of the dynamic array
};
```
下面是一个测试例子:
```cpp
int main() {
// Test constructor
CArray arr1(5);
for (int i = 0; i < arr1.length(); ++i) {
arr1.set(i, i + 1);
}
arr1.print(); // Output: 1 2 3 4 5
// Test copy constructor
CArray arr2(arr1);
arr2.print(); // Output: 1 2 3 4 5
// Test operator []
arr2[2] = 10;
arr1.print(); // Output: 1 2 3 4 5
arr2.print(); // Output: 1 2 10 4 5
// Test operator =
CArray arr3(3);
arr3 = arr2;
arr3.print(); // Output: 1 2 10
return 0;
}
```
阅读全文
相关推荐
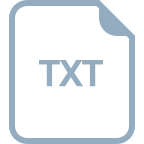
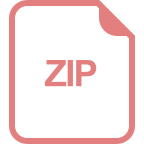
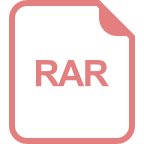















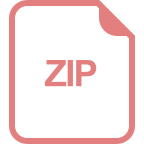