spring boot+jpa写一个一对多的增删改查
时间: 2023-05-12 14:03:57 浏览: 91
好的,以下是 Spring Boot JPA 实现一对多增删改查的示例代码:
1. 创建实体类
```
@Entity
public class Parent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Child> children = new ArrayList<>();
// getters and setters
}
@Entity
public class Child {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "parent_id")
private Parent parent;
// getters and setters
}
```
2. 创建 Repository 接口
```
public interface ParentRepository extends JpaRepository<Parent, Long> {
}
```
3. 创建 Service 类
```
@Service
public class ParentService {
@Autowired
private ParentRepository parentRepository;
public Parent save(Parent parent) {
return parentRepository.save(parent);
}
public void delete(Long id) {
parentRepository.deleteById(id);
}
public Parent findById(Long id) {
return parentRepository.findById(id).orElse(null);
}
public List<Parent> findAll() {
return parentRepository.findAll();
}
}
```
4. 创建 Controller 类
```
@RestController
@RequestMapping("/parents")
public class ParentController {
@Autowired
private ParentService parentService;
@PostMapping
public Parent create(@RequestBody Parent parent) {
return parentService.save(parent);
}
@PutMapping("/{id}")
public Parent update(@PathVariable Long id, @RequestBody Parent parent) {
Parent existingParent = parentService.findById(id);
if (existingParent == null) {
throw new RuntimeException("Parent not found");
}
existingParent.setName(parent.getName());
existingParent.setChildren(parent.getChildren());
return parentService.save(existingParent);
}
@DeleteMapping("/{id}")
public void delete(@PathVariable Long id) {
parentService.delete(id);
}
@GetMapping("/{id}")
public Parent findById(@PathVariable Long id) {
return parentService.findById(id);
}
@GetMapping
public List<Parent> findAll() {
return parentService.findAll();
}
}
```
以上就是 Spring Boot JPA 实现一对多增删改查的示例代码。
相关推荐
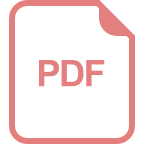
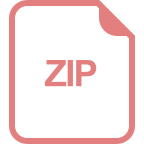
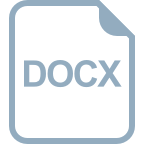














