python 正则表达式判断文件是否为excel文件
时间: 2023-06-20 14:03:28 浏览: 268
可以使用Python的re模块和os模块来判断文件名是否以“.xls”或“.xlsx”结尾。具体代码如下:
```python
import re
import os
filename = "example.xlsx"
if re.search(r'\.xls[x]?\b', filename):
print("This is an Excel file")
else:
print("This is not an Excel file")
```
其中,re.search()函数用于在字符串中查找匹配项,r'\.xls[x]?\b'是一个正则表达式,表示以“.xls”或“.xlsx”结尾的文件名,\b表示单词边界,防止误匹配其他文件名。os模块中的os.path.splitext()函数可以用来分离文件名和扩展名,比如:
```python
import os
filename = "example.xlsx"
basename, ext = os.path.splitext(filename)
if ext == ".xls" or ext == ".xlsx":
print("This is an Excel file")
else:
print("This is not an Excel file")
```
这样也可以判断文件是否为Excel文件,basename表示文件名,ext表示扩展名。
相关问题
文件夹中有以2-开头的xlsx文件,合并这些文件,每个表中有很多的续表,根据正则表达式判断续表,同时以城市为键,合并这些表格包括续表
好的,您可以使用Python中的pandas库来完成这个任务。首先,您需要使用正则表达式来筛选以2-开头的xlsx文件,并将这些文件读取到pandas的DataFrame中。然后,您可以使用正则表达式来判断哪些行是续表,并将它们添加到之前的表格中。最后,根据城市为键将所有表格合并。
以下是示例代码:
```python
import os
import re
import pandas as pd
# 正则表达式
regex = re.compile(r'^2-.*\.xlsx$')
continuation_regex = re.compile(r'^\d+\s.*$') # 这里假设续表的第一列为数字加空格
# 读取所有符合条件的xlsx文件
files = [f for f in os.listdir('.') if os.path.isfile(f) and regex.match(f)]
dfs = []
for file in files:
df = pd.read_excel(file, sheet_name=None, engine='openpyxl')
for sheet_name, sheet_df in df.items():
# 判断续表并添加到之前的表格中
if sheet_df.iloc[0, 0] and continuation_regex.match(str(sheet_df.iloc[0, 0])):
dfs[-1] = pd.concat([dfs[-1], sheet_df])
else:
dfs.append(sheet_df)
# 合并所有表格
merged_df = pd.concat(dfs)
# 根据城市为键合并所有表格
grouped_df = merged_df.groupby('城市').agg(lambda x: x.tolist())
# 将所有列表转换为字符串并重新转换为DataFrame
grouped_df = grouped_df.applymap(lambda x: ','.join([str(i) for i in x]))
grouped_df = pd.DataFrame(grouped_df.to_dict())
# 输出结果
print(grouped_df)
```
请注意,这只是示例代码,您需要根据实际情况进行调整。
创建一个空的DataFrame df = pd.DataFrame(columns=['总订单数','总推送数','推送比','问卷回馈','回馈比']) count = 0 for i in range(5): for message in messages: if "20230612统计" in message.Subject: 使用正则表达式提取订单数、推送数和回馈数的值 pattern1 = r"总订单数:(\d+)" pattern2 = r"总推送数:(\d+)" pattern3 = r"推送比:(\d+)" pattern4 = r"问卷回馈:(\d+)" pattern5 = r"回馈比:(\d+)" match1 = re.search(pattern1, message.Body) match2 = re.search(pattern2, message.Body) match3 = re.search(pattern3, message.Body) match4 = re.search(pattern4, message.Body) match5 = re.search(pattern5, message.Body) if match1 and match2 and match3 and match4 and match5: 总订单数 = match1.group(1) 总推送数 = match2.group(1) 推送比 = match3.group(1) 问卷回馈 = match4.group(1) 回馈比 = match5.group(1) print("总订单数::", 总订单数) print("总推送数:", 总推送数) print("推送比", 推送比) print("问卷回馈:", 问卷回馈) print("回馈比", 回馈比) else: print("帅哥") count += 1 if count == 1: break else: continue break df.loc[count] = ['20230612统计', message.Subject, 总订单数, 总推送数, 推送比, 问卷回馈, 回馈比] 保存DataFrame到Excel文件 df.to_excel(r'C:\Users\bjbk0026\Desktop\统计.xlsx', index=False) 推送比和回馈比设置为默认为空怎么写
如果你想在推送比和回馈比未匹配到值时将其设置为空,默认为空字符串`''`,你可以在条件判断中处理这种情况。以下是修改后的代码示例:
```python
df = pd.DataFrame(columns=['总订单数','总推送数','推送比','问卷回馈','回馈比'])
count = 0
for i in range(5):
for message in messages:
if "20230612统计" in message.Subject:
pattern1 = r"总订单数:(\d+)"
pattern2 = r"总推送数:(\d+)"
pattern3 = r"推送比:(\d+)"
pattern4 = r"问卷回馈:(\d+)"
pattern5 = r"回馈比:(\d+)"
match1 = re.search(pattern1, message.Body)
match2 = re.search(pattern2, message.Body)
match3 = re.search(pattern3, message.Body)
match4 = re.search(pattern4, message.Body)
match5 = re.search(pattern5, message.Body)
if match1 and match2 and match4:
总订单数 = match1.group(1)
总推送数 = match2.group(1)
问卷回馈 = match4.group(1)
if match3:
推送比 = match3.group(1)
else:
推送比 = '' # 设置默认为空字符串 ''
if match5:
回馈比 = match5.group(1)
else:
回馈比 = '' # 设置默认为空字符串 ''
print("总订单数::", 总订单数)
print("总推送数:", 总推送数)
print("推送比", 推送比)
print("问卷回馈:", 问卷回馈)
print("回馈比", 回馈比)
else:
print("帅哥")
count += 1
if count == 1:
break
else:
continue
break
df.loc[count] = ['20230612统计', message.Subject, 总订单数, 总推送数, 推送比, 问卷回馈, 回馈比]
df.to_excel(r'C:\Users\bjbk0026\Desktop\统计.xlsx', index=False)
```
在上述代码中,我在匹配`推送比`和`回馈比`时,使用了条件判断来处理未匹配到值的情况。如果未匹配到值,我将其设置为空字符串`''`作为默认值。然后将提取到的值添加到DataFrame中,并将DataFrame保存到Excel文件中。
希望这能满足你的需求!如果还有其他问题,请随时提问。
阅读全文
相关推荐
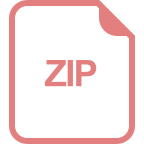
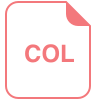
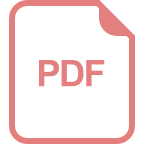
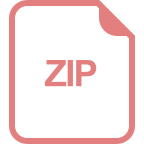
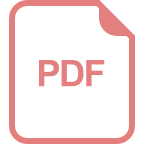
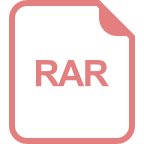
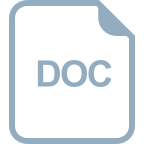
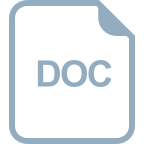
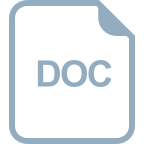
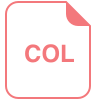
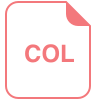



