用C语言通过栈实现输入序列的逆序输出
时间: 2023-06-04 18:08:35 浏览: 508
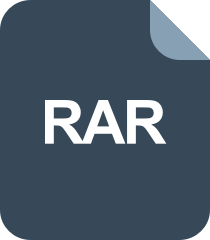
用栈实现队列逆序输出

好的,我可以回答这个问题。以下是实现输入序列逆序输出的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int x) {
if (isFull(s)) {
printf("Error: stack is full.\n");
exit(EXIT_FAILURE);
}
s->data[++(s->top)] = x;
}
int pop(Stack *s) {
if (isEmpty(s)) {
printf("Error: stack is empty.\n");
exit(EXIT_FAILURE);
}
return s->data[(s->top)--];
}
int main() {
Stack s;
int x;
initStack(&s);
while (scanf("%d", &x) == 1) {
push(&s, x);
}
while (!isEmpty(&s)) {
printf("%d ", pop(&s));
}
printf("\n");
return 0;
}
```
以上代码使用栈来实现输入序列的逆序输出,你可以通过输入一些数字来测试它。代码中的结构体 Stack 表示栈,initStack 函数用来初始化栈,isEmpty 和 isFull 函数分别用来判断栈是否为空和是否已满,push 函数用来向栈中压入一个元素,pop 函数用来弹出栈顶元素。在主函数中,我们首先初始化一个栈,然后通过循环读入一些数字,将它们依次压入栈中;最后,我们再通过循环弹出栈顶元素,并输出它们,从而实现了输入序列的逆序输出。
阅读全文
相关推荐
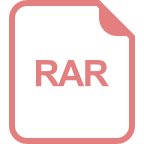















