数字影像金字塔c语言代码
时间: 2023-06-11 19:08:16 浏览: 43
数字影像金字塔可以通过递归实现,以下是一个简单的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_LEVELS 10 // 最大金字塔层数
// 读取 BMP 文件头信息
void read_bmp_header(FILE *fp, int *width, int *height) {
fseek(fp, 18, SEEK_SET);
fread(width, sizeof(int), 1, fp);
fread(height, sizeof(int), 1, fp);
}
// 读取 BMP 图像数据
void read_bmp_data(FILE *fp, unsigned char *data, int width, int height) {
int padding = (4 - (width * 3) % 4) % 4;
unsigned char pixel[3];
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
fread(pixel, sizeof(unsigned char), 3, fp);
data[i * width * 3 + j * 3 + 0] = pixel[2];
data[i * width * 3 + j * 3 + 1] = pixel[1];
data[i * width * 3 + j * 3 + 2] = pixel[0];
}
fseek(fp, padding, SEEK_CUR);
}
}
// 缩小图像
void downsample(unsigned char *src, unsigned char *dst, int src_width, int src_height, int dst_width, int dst_height) {
double scale_x = (double)src_width / dst_width;
double scale_y = (double)src_height / dst_height;
for (int i = 0; i < dst_height; i++) {
for (int j = 0; j < dst_width; j++) {
int src_x = (int)(scale_x * j);
int src_y = (int)(scale_y * i);
dst[i * dst_width * 3 + j * 3 + 0] = src[src_y * src_width * 3 + src_x * 3 + 0];
dst[i * dst_width * 3 + j * 3 + 1] = src[src_y * src_width * 3 + src_x * 3 + 1];
dst[i * dst_width * 3 + j * 3 + 2] = src[src_y * src_width * 3 + src_x * 3 + 2];
}
}
}
// 生成金字塔
void generate_pyramid(unsigned char *src, unsigned char **dst, int width, int height, int levels) {
for (int i = 0; i < levels; i++) {
dst[i] = (unsigned char *)malloc(sizeof(unsigned char) * width * height * 3);
downsample(src, dst[i], width, height, width / pow(2, i + 1), height / pow(2, i + 1));
src = dst[i];
}
}
// 保存 BMP 图像数据
void save_bmp_data(FILE *fp, unsigned char *data, int width, int height) {
int padding = (4 - (width * 3) % 4) % 4;
unsigned char pixel[3] = { 0 };
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
pixel[2] = data[i * width * 3 + j * 3 + 0];
pixel[1] = data[i * width * 3 + j * 3 + 1];
pixel[0] = data[i * width * 3 + j * 3 + 2];
fwrite(pixel, sizeof(unsigned char), 3, fp);
}
for (int j = 0; j < padding; j++) {
fwrite(&pixel, sizeof(unsigned char), 1, fp);
}
}
}
int main(int argc, char *argv[]) {
if (argc != 3) {
fprintf(stderr, "Usage: %s <input_file> <output_file>\n", argv[0]);
return 1;
}
FILE *fp_in = fopen(argv[1], "rb");
if (!fp_in) {
fprintf(stderr, "Failed to open input file %s\n", argv[1]);
return 1;
}
FILE *fp_out = fopen(argv[2], "wb");
if (!fp_out) {
fprintf(stderr, "Failed to open output file %s\n", argv[2]);
fclose(fp_in);
return 1;
}
int width, height;
read_bmp_header(fp_in, &width, &height);
unsigned char *data = (unsigned char *)malloc(sizeof(unsigned char) * width * height * 3);
read_bmp_data(fp_in, data, width, height);
unsigned char *pyramid[MAX_LEVELS];
generate_pyramid(data, pyramid, width, height, MAX_LEVELS);
for (int i = 0; i < MAX_LEVELS; i++) {
save_bmp_data(fp_out, pyramid[i], width / pow(2, i), height / pow(2, i));
}
fclose(fp_in);
fclose(fp_out);
free(data);
for (int i = 0; i < MAX_LEVELS; i++) {
free(pyramid[i]);
}
return 0;
}
```
注:本代码仅供参考,具体实现可能需要根据实际需求进行修改。
相关推荐
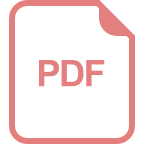














