for (int i = 0; i < list->length; i++) { cout << cur->data << " "; cur = cur->next; } cout << endl; }
时间: 2024-02-16 19:30:25 浏览: 130
这段代码看起来是遍历链表并输出每个节点的数据。但是在循环中,`i` 没有进行自增操作,这会导致无限循环。应该将 `i++` 添加到循环语句中,即 `for (int i = 0; i < list->length; i++)`。同时,还需要检查链表是否为空,以及是否存在 `cur` 指针。完整的代码可能类似于下面这样:
```c++
if (list == nullptr || list->head == nullptr) {
cout << "List is empty." << endl;
} else {
Node* cur = list->head;
for (int i = 0; i < list->length; i++) {
cout << cur->data << " ";
cur = cur->next;
}
cout << endl;
}
```
相关问题
要求如下:编写一个能为栈也能为队列的线性表,根据要求完善下列代码: #include <iostream> using namespace std; #include<string> #include"math.h" template<class T> class vlist//线性表抽象类 { public: virtual void in(T &e)=0; virtual T out()=0; }; template<class T> class Element//线性表存储元素 { public: T data; Element<T> next; Element(){} }; //在beign和end之间完成SStack和Quene类的定义及实现,其继承vlist /begin/ /end***/ int main() { vlist<int> *intp; vlist<string> *strp; SStack<int> ints; Quene<int> intq; SStack<string> strs; Quene<string> strq; intp=&ints; int t=2; intp->in(t); t=3; intp->in(t); t=4; intp->in(t); for(int i=0;i<3;i++) cout<<intp->out(); intp=&intq; t=2; intp->in(t); t=3; intp->in(t); t=4; intp->in(t); for(int i=0;i<3;i++) cout<<intp->out(); strp=&strs; string str; cout<<endl; for(int i=0;i<3;i++) { cin>>str; strp->in(str); } for(int i=0;i<3;i++) cout<<strp->out()<<" "; cout<<endl; strp=&strq; for(int i=0;i<3;i++) { cin>>str; strp->in(str); } for(int i=0;i<3;i++) cout<<strp->out()<<" "; }
代码如下:
```
#include <iostream>
#include <string>
#include <math.h>
using namespace std;
template<class T>
class vlist // 线性表抽象类
{
public:
virtual void in(T &e) = 0;
virtual T out() = 0;
};
template<class T>
class Element // 线性表存储元素
{
public:
T data;
Element<T>* next;
Element() { next = NULL; }
};
template<class T>
class SStack : public vlist<T> // 栈的实现类
{
private:
Element<T>* top; // 栈顶元素指针
public:
SStack() { top = NULL; }
void in(T &e) // 入栈操作
{
Element<T>* p = new Element<T>;
p->data = e;
p->next = top;
top = p;
}
T out() // 出栈操作
{
if (top == NULL) // 栈空,抛出异常
throw "Stack is empty!";
Element<T>* p = top;
top = top->next;
T temp = p->data;
delete p;
return temp;
}
};
template<class T>
class Quene : public vlist<T> // 队列的实现类
{
private:
Element<T>* front; // 队头元素指针
Element<T>* rear; // 队尾元素指针
public:
Quene() { front = rear = NULL; }
void in(T &e) // 入队列操作
{
Element<T>* p = new Element<T>;
p->data = e;
p->next = NULL;
if (front == NULL)
front = rear = p;
else
{
rear->next = p;
rear = p;
}
}
T out() // 出队列操作
{
if (front == NULL) // 队空,抛出异常
throw "Quene is empty!";
Element<T>* p = front;
front = front->next;
T temp = p->data;
delete p;
return temp;
}
};
int main() {
vlist<int>* intp;
vlist<string>* strp;
SStack<int> ints;
Quene<int> intq;
SStack<string> strs;
Quene<string> strq;
intp = &ints;
int t = 2;
intp->in(t);
t = 3;
intp->in(t);
t = 4;
intp->in(t);
for (int i = 0; i < 3; i++)
cout << intp->out() << " ";
intp = &intq;
t = 2;
intp->in(t);
t = 3;
intp->in(t);
t = 4;
intp->in(t);
for (int i = 0; i < 3; i++)
cout << intp->out() << " ";
strp = &strs;
string str;
cout << endl;
for (int i = 0; i < 3; i++) {
cin >> str;
strp->in(str);
}
for (int i = 0; i < 3; i++)
cout << strp->out() << " ";
cout << endl;
strp = &strq;
for (int i = 0; i < 3; i++) {
cin >> str;
strp->in(str);
}
for (int i = 0; i < 3; i++)
cout << strp->out() << " ";
}
```
这段代码定义了一个 vlist 抽象类,并且定义了两个线性表的实现类:SStack 和 Quene,分别实现了栈和队列的基本操作。在 main 函数中,分别使用了栈和队列的实现类,演示了它们的基本操作。
cout << cur->next->val << " ";
cout << cur->next->val << " ";是C++中的输出语句,使用<<符号将变量输出到控制台上。其中,cur是一个指针,它指向的是一个链表中的某个节点,cur->next表示当前节点的下一个节点,而cur->next->val则表示下一个节点的值。这条语句的作用是输出当前节点的下一个节点的值,并在后面加上一个空格。
阅读全文
相关推荐
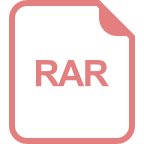
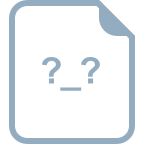
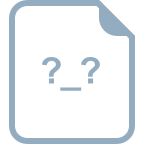












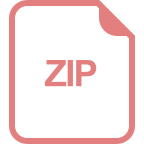
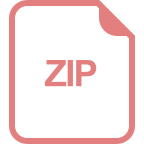