android三个骰子摇动动画,【Android】OpenGL ES实现3D抛骰子
时间: 2024-02-05 19:13:00 浏览: 26
实现三个骰子的摇动动画可以使用Android的动画系统,具体步骤如下:
1. 首先定义三个ImageView控件,分别表示三个骰子。
2. 在res目录下创建一个anim文件夹,用于存放动画文件。
3. 在anim文件夹下创建三个xml文件,分别表示三个骰子的摇动动画。
4. 编辑每个xml文件,实现骰子的摇动动画,例如:
```xml
<!-- 骰子1的摇动动画 -->
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/accelerate_interpolator">
<rotate
android:duration="100"
android:fromDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:repeatCount="5"
android:repeatMode="reverse"
android:toDegrees="30" />
<rotate
android:duration="100"
android:fromDegrees="30"
android:pivotX="50%"
android:pivotY="50%"
android:repeatCount="5"
android:repeatMode="reverse"
android:toDegrees="-30" />
<rotate
android:duration="100"
android:fromDegrees="-30"
android:pivotX="50%"
android:pivotY="50%"
android:repeatCount="5"
android:repeatMode="reverse"
android:toDegrees="0" />
</set>
```
5. 在代码中使用AnimationUtils加载动画,并将动画应用到每个ImageView控件上,例如:
```java
// 加载动画文件
Animation anim1 = AnimationUtils.loadAnimation(this, R.anim.anim_dice1);
Animation anim2 = AnimationUtils.loadAnimation(this, R.anim.anim_dice2);
Animation anim3 = AnimationUtils.loadAnimation(this, R.anim.anim_dice3);
// 应用动画到ImageView控件
ImageView dice1 = findViewById(R.id.dice1);
ImageView dice2 = findViewById(R.id.dice2);
ImageView dice3 = findViewById(R.id.dice3);
dice1.startAnimation(anim1);
dice2.startAnimation(anim2);
dice3.startAnimation(anim3);
```
至此,就可以实现三个骰子的摇动动画。
如果需要使用OpenGL ES实现3D抛骰子,可以参考以下步骤:
1. 在res目录下创建一个raw文件夹,用于存放骰子模型的obj文件和材质文件。
2. 使用OpenGL ES加载骰子模型,可以使用第三方库如OBJParser或者自行编写解析代码。
3. 实现骰子的抛掷动画,可以使用随机数生成每个骰子的旋转角度,然后使用OpenGL ES的旋转功能实现。
4. 实现骰子的投掷效果,可以使用物理引擎如Bullet Physics或者自行编写物理模拟代码。
5. 在SurfaceView中绘制骰子模型,并在onDrawFrame方法中更新骰子的位置和旋转角度。
相关推荐
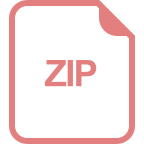














