用java 写出以下代码:日期类使用三个整数记录年、月、日,其中月份的取值范围为1-12,日期的取值范围为1-31,尝试写出该类的构造函数,接受三个整数作为年月日的值,并验证月份和日期的有效性,当给出的日期数据无效时抛出自定义的日期范围异常,并写出测试代码,从不同的角度触发异常。
时间: 2023-11-23 14:05:41 浏览: 115
好的,我来写出相关的代码:
```java
public class Date {
private int year;
private int month;
private int day;
public Date(int year, int month, int day) throws InvalidDateException {
if (year < 0 || month < 1 || month > 12 || day < 1 || day > 31) {
throw new InvalidDateException("Invalid date range");
}
if ((month == 4 || month == 6 || month == 9 || month == 11) && day > 30) {
throw new InvalidDateException("Invalid date range");
}
if (month == 2 && day > 29) {
throw new InvalidDateException("Invalid date range");
}
if (month == 2 && day == 29 && !isLeapYear(year)) {
throw new InvalidDateException("Invalid date range");
}
this.year = year;
this.month = month;
this.day = day;
}
private boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
// getters and setters
public static void main(String[] args) {
try {
Date d1 = new Date(2022, 2, 29); // invalid date
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
Date d2 = new Date(-2022, 2, 28); // invalid date
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
Date d3 = new Date(2022, 13, 31); // invalid date
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
Date d4 = new Date(2022, 4, 31); // invalid date
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
Date d5 = new Date(2021, 2, 29); // invalid date
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
Date d6 = new Date(2024, 2, 29); // valid date
System.out.println(d6.getYear() + "-" + d6.getMonth() + "-" + d6.getDay());
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
}
}
class InvalidDateException extends Exception {
public InvalidDateException(String message) {
super(message);
}
}
```
上面的代码中,我们定义了一个 `Date` 类来表示日期,使用年、月、日三个整数来记录日期。在构造函数中,我们首先对年、月、日的有效性进行了验证,如果不在有效范围内,则抛出自定义的 `InvalidDateException` 异常。在验证月份和日期有效性时,我们还需要特别注意闰年的情况,因为二月份的天数会受到闰年的影响。
在测试代码中,我们分别尝试使用不同的参数来创建 `Date` 对象,有些参数是有效的,有些是无效的。通过这些测试,我们可以从不同的角度来验证异常的触发情况。
阅读全文
相关推荐






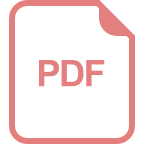









