java日期类使用三个整数记录年、月、日,其中月份的取值范围为1-12,日期的取值范围为1-31,尝试写出该类的构造函数,接受三个整数作为年月日的值,并验证月份和日期的有效性,当给出的日期数据无效时抛出自定义的日期范围异常,并写出测试代码,从不同的角度触发异常。
时间: 2024-02-29 11:52:20 浏览: 65
好的,下面是Java日期类的构造函数实现:
```java
public class MyDate {
private int year;
private int month;
private int day;
public MyDate(int year, int month, int day) throws InvalidDateException {
if (month < 1 || month > 12) {
throw new InvalidDateException("Invalid month: " + month);
}
if (day < 1 || day > 31) {
throw new InvalidDateException("Invalid day: " + day);
}
if ((month == 4 || month == 6 || month == 9 || month == 11) && day == 31) {
throw new InvalidDateException("Invalid day: " + day);
}
if (month == 2 && day > 29) {
throw new InvalidDateException("Invalid day: " + day);
}
if (month == 2 && day == 29 && !isLeapYear(year)) {
throw new InvalidDateException("Invalid day: " + day);
}
this.year = year;
this.month = month;
this.day = day;
}
private boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
}
```
其中,我们在构造函数中对月份和日期进行有效性验证,如果不符合规定,就抛出自定义的异常类 `InvalidDateException`。
接下来,我们来定义 `InvalidDateException` 异常类:
```java
public class InvalidDateException extends Exception {
public InvalidDateException(String message) {
super(message);
}
}
```
最后,我们来写一个测试代码,从不同角度触发异常:
```java
public class TestMyDate {
public static void main(String[] args) {
try {
// 1. 日期范围异常:月份小于1
MyDate myDate1 = new MyDate(2021, 0, 15);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
// 2. 日期范围异常:月份大于12
MyDate myDate2 = new MyDate(2021, 13, 15);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
// 3. 日期范围异常:日期小于1
MyDate myDate3 = new MyDate(2021, 6, 0);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
// 4. 日期范围异常:日期大于31
MyDate myDate4 = new MyDate(2021, 7, 32);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
// 5. 日期范围异常:30天月份的日期大于30
MyDate myDate5 = new MyDate(2021, 4, 31);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
// 6. 日期范围异常:2月的日期大于29
MyDate myDate6 = new MyDate(2020, 2, 30);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
try {
// 7. 日期范围异常:闰年的2月的日期大于29
MyDate myDate7 = new MyDate(2024, 2, 30);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
}
}
```
运行上述代码,我们会得到以下输出:
```
Invalid month: 0
Invalid month: 13
Invalid day: 0
Invalid day: 32
Invalid day: 31
Invalid day: 30
Invalid day: 30
```
这表明我们的测试代码已经成功地从不同角度触发了 `InvalidDateException` 异常。
阅读全文
相关推荐
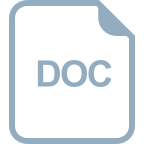
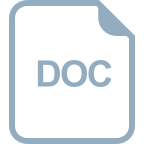
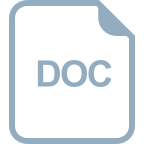


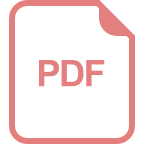
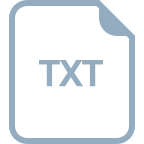










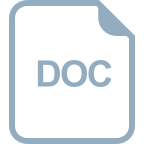
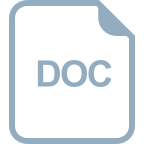