用Python 解决数独
时间: 2023-11-16 10:17:52 浏览: 111
可以使用回溯算法来解决数独问题,以下是一个用Python实现的数独解决器:
```python
def solve_sudoku(board):
"""
Solves a given Sudoku board.
:param board: a 9x9 list of integers representing the Sudoku board
:return: True if the board is solvable, False otherwise
"""
# Find the next empty cell
row, col = find_empty_cell(board)
# If there are no empty cells, the board must be solved
if row is None:
return True
# Try every possible number for the empty cell
for num in range(1, 10):
if is_valid_move(board, row, col, num):
board[row][col] = num
# Recursively solve the rest of the board
if solve_sudoku(board):
return True
# If the recursive call did not find a solution, backtrack
board[row][col] = 0
# If no number works for the empty cell, the board is unsolvable
return False
def find_empty_cell(board):
"""
Finds the first empty cell on the board.
:param board: a 9x9 list of integers representing the Sudoku board
:return: a tuple (row, col) of the first empty cell, or None if the board is full
"""
for row in range(9):
for col in range(9):
if board[row][col] == 0:
return row, col
return None, None
def is_valid_move(board, row, col, num):
"""
Checks if a given move is valid for the given board.
:param board: a 9x9 list of integers representing the Sudoku board
:param row: the row of the cell being filled
:param col: the column of the cell being filled
:param num: the number being placed in the cell
:return: True if the move is valid, False otherwise
"""
# Check the row
if num in board[row]:
return False
# Check the column
if num in [board[i][col] for i in range(9)]:
return False
# Check the 3x3 square
square_row = (row // 3) * 3
square_col = (col // 3) * 3
if num in [board[i][j] for i in range(square_row, square_row + 3) for j in range(square_col, square_col + 3)]:
return False
# If all checks pass, the move is valid
return True
```
你可以将一个数独题目表示成一个9x9的列表,其中空白格子用0表示。例如,下面是一个数独题目的表示:
```
[
[5, 3, 0, 0, 7, 0, 0, 0, 0],
[6, 0, 0, 1, 9, 5, 0, 0, 0],
[0, 9, 8, 0, 0, 0, 0, 6, 0],
[8, 0, 0, 0, 6, 0, 0, 0, 3],
[4, 0, 0, 8, 0, 3, 0, 0, 1],
[7, 0, 0, 0, 2, 0, 0, 0, 6],
[0, 6, 0, 0, 0, 0, 2, 8, 0],
[0, 0, 0, 4, 1, 9, 0, 0, 5],
[0, 0, 0, 0, 8, 0, 0, 7, 9]
]
```
调用`solve_sudoku`函数将会在原地修改这个列表,使其变成数独的解(如果有解)。如果数独无解,函数将返回False。
阅读全文
相关推荐
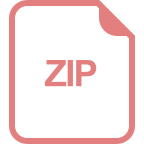

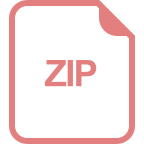
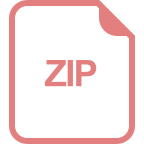
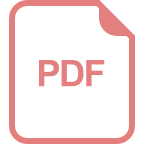
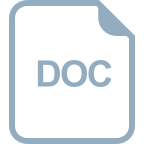
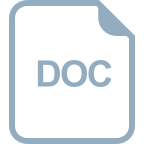
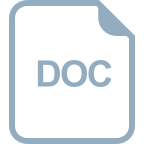
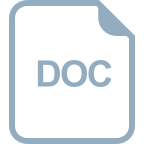
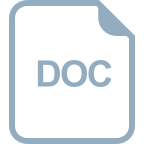




