如何用python根据地址查找经纬度
时间: 2024-04-05 21:29:51 浏览: 27
你可以使用Python中的geopy库来根据地址查找经纬度。以下是一个简单的例子:
```python
from geopy.geocoders import Nominatim
address = "北京市海淀区中关村大街27号"
geolocator = Nominatim(user_agent="my_app") # 创建geolocator对象
location = geolocator.geocode(address) # 根据地址查找经纬度
print((location.latitude, location.longitude)) # 打印经纬度
```
在这个例子中,我们首先导入geopy库中的Nominatim类,然后创建了一个geolocator对象。接下来,我们使用geolocator对象的geocode方法来查找指定地址的经纬度,并将结果存储在location变量中。最后,我们打印了location的latitude和longitude属性,分别对应查找到的纬度和经度。
相关问题
如何用python根据地址查找经纬度提供多个方法
除了使用geopy库之外,还可以使用其他的Python库来根据地址查找经纬度。以下是一些常用的方法:
1. 使用百度地图API
百度地图API提供了根据地址查找经纬度的服务,可以通过发送HTTP请求获取结果。具体操作步骤如下:
- 在百度地图开放平台上注册账号,并创建应用,获取API key。
- 通过发送HTTP请求获取结果,示例代码如下:
```python
import requests
def get_location_by_baidu(address, ak):
url = f"http://api.map.baidu.com/geocoding/v3/?address={address}&output=json&ak={ak}"
response = requests.get(url)
json_data = response.json()
if json_data['status'] == 0:
return json_data['result']['location']['lat'], json_data['result']['location']['lng']
else:
return None
```
其中,`address` 是要查询的地址,`ak` 是从百度地图开放平台获取的API key。
2. 使用高德地图API
高德地图API也提供了根据地址查找经纬度的服务,可以通过发送HTTP请求获取结果。具体操作步骤如下:
- 在高德开放平台上注册账号,并创建应用,获取API key。
- 通过发送HTTP请求获取结果,示例代码如下:
```python
import requests
def get_location_by_gaode(address, key):
url = f"https://restapi.amap.com/v3/geocode/geo?key={key}&address={address}"
response = requests.get(url)
json_data = response.json()
if json_data['status'] == '1' and int(json_data['count']) > 0:
location = json_data['geocodes'][0]['location'].split(',')
return location[1], location[0]
else:
return None
```
其中,`address` 是要查询的地址,`key` 是从高德开放平台获取的API key。
3. 使用腾讯地图API
腾讯地图API也提供了根据地址查找经纬度的服务,可以通过发送HTTP请求获取结果。具体操作步骤如下:
- 在腾讯云开发者平台上注册账号,并创建应用,获取密钥。
- 通过发送HTTP请求获取结果,示例代码如下:
```python
import requests
def get_location_by_tencent(address, key):
url = f"https://apis.map.qq.com/ws/geocoder/v1/?address={address}&key={key}"
response = requests.get(url)
json_data = response.json()
if json_data['status'] == 0:
location = json_data['result']['location']
return location['lat'], location['lng']
else:
return None
```
其中,`address` 是要查询的地址,`key` 是从腾讯云开发者平台获取的密钥。
如何用python根据地址批量查找经纬度
要使用Python根据地址批量查找经纬度,可以使用Geopy库。以下是一个示例代码,可以从CSV文件中读取地址,使用Geopy库查找经纬度,然后将结果写入新的CSV文件中:
```python
import csv
from geopy.geocoders import Nominatim
geolocator = Nominatim(user_agent="my-application")
# Open the input and output CSV files
with open('input.csv', 'r') as infile, open('output.csv', 'w') as outfile:
reader = csv.reader(infile)
writer = csv.writer(outfile)
# Loop through each row in the input CSV file
for row in reader:
address = row[0] # The address to lookup
# Use Geopy to lookup the latitude and longitude for this address
location = geolocator.geocode(address)
# Write the results to the output CSV file
if location is not None:
lat = location.latitude
lon = location.longitude
writer.writerow([address, lat, lon])
else:
writer.writerow([address, "", ""])
```
在这个示例中,我们首先导入了`csv`和`Nominatim`类。`Nominatim`是Geopy库中用于查找地理位置信息的类。然后我们打开输入和输出CSV文件。我们使用`csv.reader()`来读取输入文件的每一行,并使用`csv.writer()`来将结果写入输出文件。
在循环中,我们读取每一行的地址,然后使用`geolocator.geocode()`方法从Geopy库中查找该地址的经纬度。如果找到了地址,则将其写入输出CSV文件中。如果无法找到地址,则将空的经纬度写入输出文件中。
要运行此代码,请将输入CSV文件名更改为您的文件名,并将输出CSV文件名更改为您要创建的文件名。然后,运行代码即可。
阅读全文
相关推荐
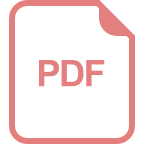












