不使用threadlocal的前提下将用户上下文信息作为参数传递到Spring AOP的切面中然后在需要的方法中获取具体该怎么做?
时间: 2024-02-11 10:06:52 浏览: 245
如果不使用ThreadLocal,将用户上下文信息作为参数传递到Spring AOP的切面中,可以通过以下步骤实现:
1. 定义一个用于保存用户上下文信息的类,例如:
```
public class UserContext {
private String userId;
private String userName;
// 省略getter和setter方法
}
```
2. 在Spring AOP的切面中定义一个`UserContext`类型的参数,并使用`@Around`注解将该参数绑定到切点上。例如:
```
@Aspect
public class UserContextAspect {
@Around("execution(* com.example.demo.service..*(..)) && args(.., userContext)")
public Object around(ProceedingJoinPoint joinPoint, UserContext userContext) throws Throwable {
try {
// 在当前线程上下文中保存用户上下文信息
UserContext.setCurrentUserContext(userContext);
// 执行目标方法
return joinPoint.proceed();
} finally {
// 清除当前线程上下文中的用户上下文信息
UserContext.removeCurrentUserContext();
}
}
}
```
在上面的代码中,`@Around`注解定义了一个环绕通知,用于将传入的用户上下文信息保存到当前线程上下文中,并将该参数绑定到切点上。在方法调用前,将用户上下文信息保存到当前线程上下文中;在方法调用后,清除当前线程上下文中的用户上下文信息。
3. 在需要使用用户上下文信息的方法中,添加一个`UserContext`类型的参数,并在调用该方法时传递当前线程的用户上下文信息。例如:
```
@Service
public class UserService {
public User getById(Long id, UserContext userContext) {
// 从当前线程上下文中获取用户上下文信息
String userId = userContext.getUserId();
String userName = userContext.getUserName();
// 根据用户ID查询用户信息
User user = userDao.getById(id);
// TODO: 根据用户上下文信息进行业务处理
return user;
}
}
```
在上面的代码中,`getById`方法添加了一个`UserContext`类型的参数,并在业务逻辑中使用该参数获取当前线程的用户上下文信息,然后根据该信息进行业务处理。
通过以上步骤,就可以将用户上下文信息作为参数传递到Spring AOP的切面中,并在需要使用该信息的方法中获取。需要注意的是,在调用方法时需要手动传递用户上下文信息,否则会出现空指针异常。另外,需要在当前线程上下文中保存用户上下文信息和清除用户上下文信息,否则会出现数据混乱的问题。
阅读全文
相关推荐
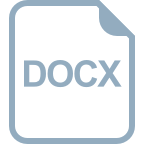
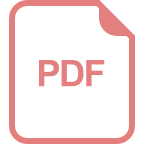
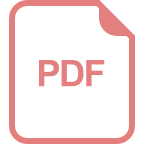
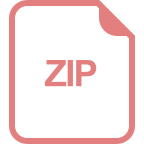
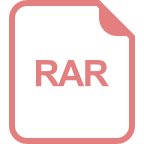
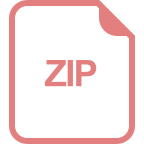
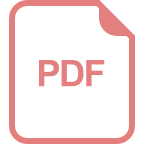
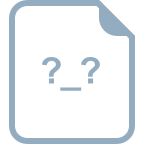
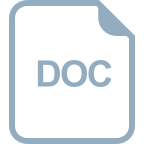
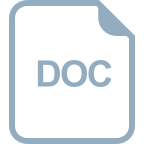
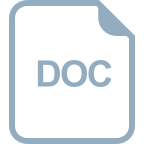
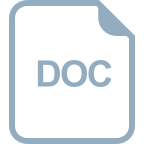
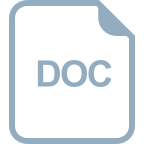
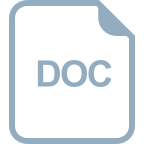
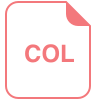
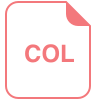
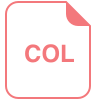