最炫酷的Python代码
时间: 2023-11-05 13:28:00 浏览: 92
Python有很多有趣的代码,以下是一个简单的例子,可以在命令行中以彩色模式打印出“Hello World!”:
```python
import colorama
from colorama import Fore, Back, Style
colorama.init(autoreset=True)
print(Fore.BLUE + Back.YELLOW + "Hello World!")
print(Back.CYAN + "I am a Python AI assistant." + Style.RESET_ALL)
```
这段代码使用了colorama库,将文本变成了蓝色的字体和黄色的背景。第二行代码初始化colorama库,以便在Windows上正常工作。第4行代码打印出“Hello World!”并使用前景色和背景色。第5行代码打印出一个带有CYAN背景颜色的字符串。
相关问题
炫酷python代码烟花
Python 中炫酷的烟花效果通常通过图形库如 Pygame 或者更高级的绘图模块如 P5.py 来实现。下面是一个简单的使用 Pygame 创建动画烟花的例子:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小
win_size = (800, 600)
screen = pygame.display.set_mode(win_size)
# 烟花颜色和速度范围
colors = [(255, 0, 0), (0, 255, 0), (0, 0, 255)]
speeds = [random.randint(1, 5) for _ in range(3)]
# 主循环
while True:
# 清除屏幕
screen.fill((0, 0, 0))
# 遍历烟花
for i, color in enumerate(colors):
# 更新位置并绘制
pos_x, pos_y = random.uniform(-win_size[0]/2, win_size[0]/2), random.uniform(-win_size[1]/2, win_size[1]/2)
size = speeds[i] * 2 + 1
pygame.draw.circle(screen, color, (int(pos_x), int(pos_y)), size)
# 更新显示
pygame.display.update()
# 检查事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 控制烟花的更新频率
pygame.time.delay(100)
```
这个代码会创建一些随机位置、颜色和大小的圆点,模拟烟花在屏幕上绽放的效果。你可以根据需要调整颜色列表、速度范围等参数来制作不同的烟花效果。
Python炫酷烟花代码
在Python中,我们可以利用Pygame库和一些基础的数学原理来编写一个简单的烟花动画效果,它通常涉及到随机生成位置、颜色和大小的像素点。下面是一个简化的例子:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Python烟花")
# 烟花粒子的颜色和速度范围
colors = [(255, 0, 0), (0, 255, 0), (0, 0, 255)]
velocities = [(-3, -4), (-2, 2), (3, 4)]
# 游戏主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 清空屏幕
screen.fill((0, 0, 0))
# 创建新的烟花
particles = []
for _ in range(random.randint(1, 10)):
x = random.randint(0, screen.get_width())
y = random.randint(0, screen.get_height())
color = random.choice(colors)
velocity = random.choice(velocities)
particles.append([x, y, color, velocity])
# 绘制烟花
for particle in particles:
x, y, color, vel = particle
pygame.draw.circle(screen, color, (x, y), random.randint(10, 50))
x += vel[0]
y += vel[1]
if x < 0 or x > screen.get_width() or y < 0 or y > screen.get_height():
velocities.remove(vel)
particle[0] = x
particle[1] = y
# 更新屏幕
pygame.display.flip()
```
这个代码会创建一个不断发射并移动的简单烟花效果。每个烟花由一个小圆圈表示,颜色和运动路径都是随机的。
阅读全文
相关推荐
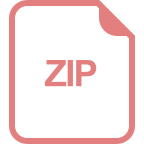
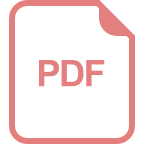
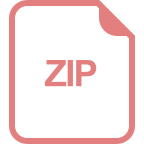












