C#判断一个文件夹是否存在,不存在新建
时间: 2023-06-21 08:22:50 浏览: 233
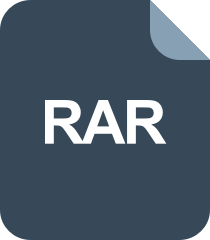
C#判断文件及文件夹是否存在并创建

您可以使用C#中的Directory类来判断文件夹是否存在,并用Directory.CreateDirectory()方法来创建新文件夹。以下是示例代码:
```csharp
using System.IO;
string folderPath = @"C:\example\folder";
if (!Directory.Exists(folderPath))
{
Directory.CreateDirectory(folderPath);
}
```
在上面的示例中,我们定义了一个文件夹路径`folderPath`,然后使用Directory.Exists()方法来检查该文件夹是否存在。如果不存在,就使用Directory.CreateDirectory()方法来创建新的文件夹。
阅读全文
相关推荐

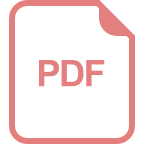

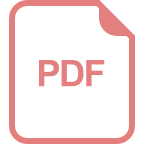
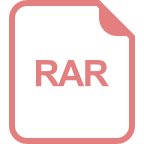
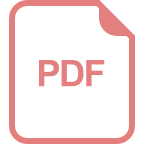
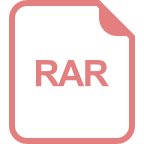
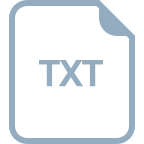
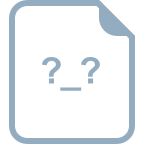
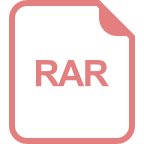
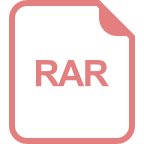
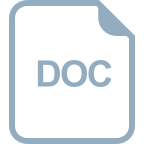


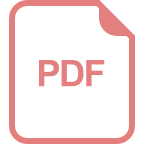