使用pytorch编写一个带有注意力机制的bp神经网络分类模型,输入为4维特征,输出3类
时间: 2023-05-16 08:07:43 浏览: 76
非常感谢您的提问。以下是使用 PyTorch 编写带有注意力机制的 BP 神经网络分类模型的示例代码:
```python
import torch
import torch.nn as nn
class Attention(nn.Module):
def __init__(self, hidden_size):
super(Attention, self).__init__()
self.hidden_size = hidden_size
self.attn = nn.Linear(self.hidden_size * 2, hidden_size)
self.v = nn.Linear(hidden_size, 1, bias=False)
def forward(self, hidden, encoder_outputs):
max_len = encoder_outputs.size(0)
batch_size = encoder_outputs.size(1)
# Repeat hidden state max_len times
hidden = hidden.unsqueeze(1).repeat(1, max_len, 1)
# Concatenate hidden state and encoder outputs
energy = torch.tanh(self.attn(torch.cat((hidden, encoder_outputs), dim=2)))
# Calculate attention weights
attention = self.v(energy).squeeze(2)
attention = torch.softmax(attention, dim=1)
# Calculate context vector
context = torch.bmm(attention.unsqueeze(1), encoder_outputs.transpose(0, 1))
context = context.squeeze(1)
return context, attention
class Classifier(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(Classifier, self).__init__()
self.hidden_size = hidden_size
self.embedding = nn.Linear(input_size, hidden_size)
self.gru = nn.GRU(hidden_size, hidden_size)
self.attention = Attention(hidden_size)
self.out = nn.Linear(hidden_size, output_size)
def forward(self, input):
embedded = self.embedding(input)
output, hidden = self.gru(embedded)
context, attention = self.attention(hidden, output)
output = self.out(context)
return output, attention
# Define model
model = Classifier(input_size=4, hidden_size=64, output_size=3)
```
此模型使用了 PyTorch 中的 nn.Module 类,包含了一个带有注意力机制的 GRU 神经网络。输入为 4 维特征,输出为 3 类。注意力机制可以帮助模型更好地关注输入中的重要信息,提高分类准确率。
希望这个示例代码能够帮助您。如果您有任何问题,请随时问我。
相关推荐
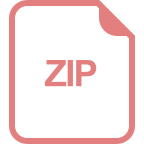
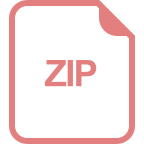
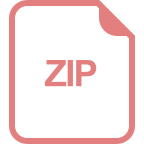









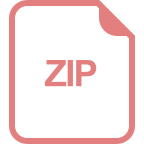
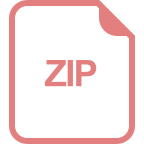
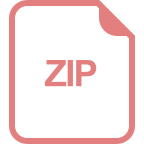
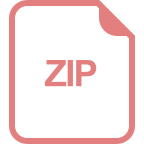
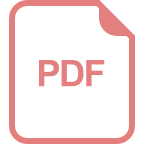