输入一个句子,用C语言编写一个函数,它的输入就是这个句子,把这个句子中的小写字母 变成大写字母,把大写字母变成小写字母,并在屏幕上打印出来。
时间: 2024-05-10 08:20:00 浏览: 33
```c
#include <stdio.h>
#include <ctype.h>
void transform(char* sentence){
int i = 0;
while(sentence[i] != '\0'){
if(islower(sentence[i])){
sentence[i] = toupper(sentence[i]);
}else if(isupper(sentence[i])){
sentence[i] = tolower(sentence[i]);
}
i++;
}
printf("%s\n", sentence);
}
int main(){
char sentence[] = "Hello World!";
transform(sentence);
return 0;
}
```
相关问题
输入一个句子,用C语言编写一个函数,不使用ctype.h,它的输入就是这个句子,把这个句子中的小写字母 变成大写字母,把大写字母变成小写字母,并在屏幕上打印出来。
```c
#include <stdio.h>
void changeCase(char sentence[]) {
int i = 0;
while (sentence[i] != '\0') {
if (sentence[i] >= 'a' && sentence[i] <= 'z') {
sentence[i] = sentence[i] - 32;
}
else if (sentence[i] >= 'A' && sentence[i] <= 'Z') {
sentence[i] = sentence[i] + 32;
}
i++;
}
printf("%s", sentence);
}
int main() {
char sentence[100];
printf("Please enter a sentence: ");
fgets(sentence, 100, stdin);
changeCase(sentence);
return 0;
}
```
编写用c语言编写一个函数,可以把单个的大写字母转换为小写字母。在main函数中完成输入和输出。
```c
#include <stdio.h>
char toLower(char c) {
if (c >= 'A' && c <= 'Z') {
return c + ('a' - 'A');
}
return c;
}
int main() {
char c;
printf("请输入一个大写字母:");
scanf("%c", &c);
printf("转换后的小写字母为:%c\n", toLower(c));
return 0;
}
```
阅读全文
相关推荐
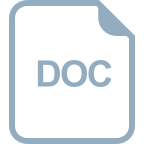
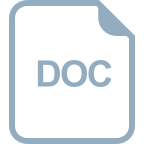
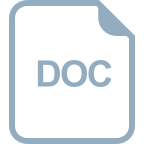













