在C/C++开发一个计算器程序,实现加减乘除四则运算和括号和嵌套计算器,支
时间: 2023-12-03 13:43:11 浏览: 59
持多位数输入和小数计算。可以使用栈来实现括号和嵌套计算器。
以下是一个简单的示例代码:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断字符是否为数字
bool isDigit(char c) {
return c >= '0' && c <= '9';
}
// 将字符串转化为数字
double strToDouble(string str) {
double res = 0.0;
double base = 1.0;
bool flag = false; // 是否有小数点
int i = 0;
if (str[i] == '-') {
flag = true;
++i;
}
for (; i < str.size(); ++i) {
if (str[i] == '.') {
flag = true;
continue;
}
if (flag) {
base *= 0.1;
res += (str[i] - '0') * base;
} else {
res = res * 10 + (str[i] - '0');
}
}
return flag ? (flag && str[0] == '-' ? -res : res) : (flag && str[0] == '-' ? -res : res);
}
// 计算两个数的运算结果
double calculate(double a, double b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
default: return 0;
}
}
// 计算表达式的值
double evaluate(string expr) {
stack<double> nums;
stack<char> ops;
for (int i = 0; i < expr.size(); ++i) {
if (isDigit(expr[i])) { // 数字
int j = i;
while (j < expr.size() && (isDigit(expr[j]) || expr[j] == '.')) {
++j;
}
double num = strToDouble(expr.substr(i, j - i));
nums.push(num);
i = j - 1;
} else if (expr[i] == '(') { // 左括号
ops.push(expr[i]);
} else if (expr[i] == ')') { // 右括号
while (ops.top() != '(') {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
ops.pop();
} else if (expr[i] == '+' || expr[i] == '-') { // 加减
while (!ops.empty() && ops.top() != '(') {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
ops.push(expr[i]);
} else if (expr[i] == '*' || expr[i] == '/') { // 乘除
while (!ops.empty() && (ops.top() == '*' || ops.top() == '/')) {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
ops.push(expr[i]);
}
}
while (!ops.empty()) {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
return nums.top();
}
int main() {
string expr;
cout << "请输入表达式:" << endl;
cin >> expr;
double res = evaluate(expr);
cout << "计算结果为:" << res << endl;
return 0;
}
```
这个程序支持多位数输入和小数计算,可以通过栈来实现括号和嵌套计算器。
相关推荐
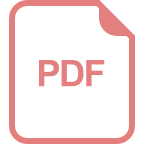














