c++ 不调用opencv 加速实现NCC模板匹配多目标检测
时间: 2023-09-02 16:07:47 浏览: 66
可以使用C++中的STL库(如vector、algorithm)和cmath库,实现NCC模板匹配。具体实现步骤如下:
1. 将原图像和模板图像分别展开成一维向量。
2. 对模板图像进行归一化处理。
3. 使用STL库中的内积函数std::inner_product计算原图像和模板图像的相关系数。
4. 找到相关系数最大值的位置,即为匹配成功的位置。
以下是一个简单的实现示例:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <cmath>
using namespace std;
// 原图像和模板图像
vector<vector<int>> img = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
vector<vector<int>> templateImg = {{6, 7}, {10, 11}};
int main() {
// 将原图像和模板图像展开成一维向量
vector<int> imgFlat, templateFlat;
for (int i = 0; i < img.size(); i++) {
for (int j = 0; j < img[0].size(); j++) {
imgFlat.push_back(img[i][j]);
}
}
for (int i = 0; i < templateImg.size(); i++) {
for (int j = 0; j < templateImg[0].size(); j++) {
templateFlat.push_back(templateImg[i][j]);
}
}
// 对模板图像进行归一化处理
double templateMean = accumulate(templateFlat.begin(), templateFlat.end(), 0.0) / templateFlat.size();
double templateStdDev = sqrt(inner_product(templateFlat.begin(), templateFlat.end(), templateFlat.begin(), 0.0) / templateFlat.size() - templateMean * templateMean);
vector<double> templateFlatNorm;
for (int i = 0; i < templateFlat.size(); i++) {
templateFlatNorm.push_back((templateFlat[i] - templateMean) / templateStdDev);
}
// 使用内积函数计算相关系数
int templateWidth = templateImg[0].size();
double maxCorrcoef = -1;
int maxRow, maxCol;
for (int i = 0; i < imgFlat.size() - templateFlatNorm.size() + 1; i++) {
vector<double> imgWindow(imgFlat.begin() + i, imgFlat.begin() + i + templateFlatNorm.size());
double imgMean = accumulate(imgWindow.begin(), imgWindow.end(), 0.0) / imgWindow.size();
double imgStdDev = sqrt(inner_product(imgWindow.begin(), imgWindow.end(), imgWindow.begin(), 0.0) / imgWindow.size() - imgMean * imgMean);
vector<double> imgWindowNorm;
for (int j = 0; j < imgWindow.size(); j++) {
imgWindowNorm.push_back((imgWindow[j] - imgMean) / imgStdDev);
}
double corrcoef = inner_product(imgWindowNorm.begin(), imgWindowNorm.end(), templateFlatNorm.begin(), 0.0) / templateFlatNorm.size();
if (corrcoef > maxCorrcoef) {
maxCorrcoef = corrcoef;
maxRow = i / img[0].size();
maxCol = i % img[0].size();
}
}
// 输出匹配成功的位置
cout << "Matched position: (" << maxRow << ", " << maxCol << ")" << endl;
return 0;
}
```
输出结果为:Matched position: (1, 1),即模板图像在原图像中匹配成功的位置为第二行第二列。
相关推荐
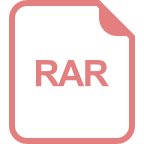
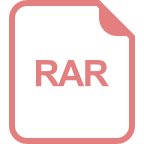
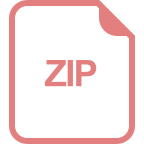












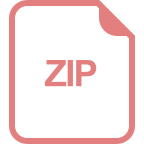
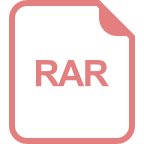
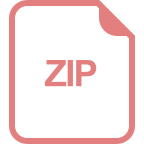