spingboot 如何集成 jsch
时间: 2023-03-21 09:02:47 浏览: 105
要在Spring Boot应用程序中集成JSch库,您需要执行以下步骤:
1. 将JSch库添加到您的应用程序依赖项中。您可以将以下依赖项添加到您的pom.xml文件中:
```xml
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.55</version>
</dependency>
```
2. 在您的应用程序中创建一个Spring Bean,该Bean将作为JSch会话的工厂。您可以使用以下代码创建此Bean:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
@Configuration
public class JSchConfiguration {
@Bean
public Session jschSession() throws JSchException {
JSch jsch = new JSch();
Session session = jsch.getSession("username", "hostname", 22);
session.setConfig("StrictHostKeyChecking", "no");
session.setPassword("password");
session.connect();
return session;
}
}
```
请注意,在上面的示例中,我们创建了一个名为`jschSession()`的Spring Bean,该Bean返回一个连接到远程主机的JSch会话。
3. 在需要使用JSch会话的任何Spring Bean中注入JSch会话Bean。例如,如果您需要在某个Spring Service类中使用JSch会话,则可以使用以下代码注入该Bean:
```java
import org.springframework.stereotype.Service;
import com.jcraft.jsch.Session;
@Service
public class MyService {
private final Session session;
public MyService(Session session) {
this.session = session;
}
// ... other methods that use the JSch session ...
}
```
在上面的示例中,我们将JSch会话Bean注入了MyService类中。
现在,您可以在任何Spring Bean中使用JSch会话来执行SSH命令或传输文件。例如,您可以使用以下代码在远程主机上执行命令:
```java
import com.jcraft.jsch.ChannelExec;
import com.jcraft.jsch.Session;
@Service
public class MyService {
private final Session session;
public MyService(Session session) {
this.session = session;
}
public void executeCommand(String command) throws JSchException {
ChannelExec channel = (ChannelExec) session.openChannel("exec");
channel.setCommand(command);
channel.connect();
// read the command output here...
channel.disconnect();
}
}
```
在上面的示例中,我们使用JSch会话执行了一个命令,并读取了命令的输出。
阅读全文
相关推荐
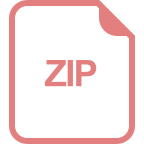
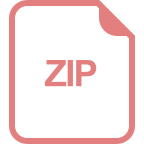
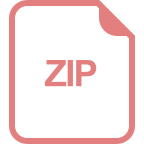





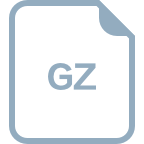
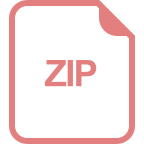
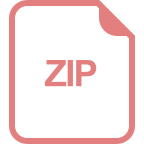


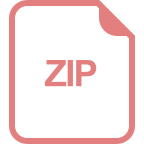
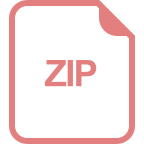
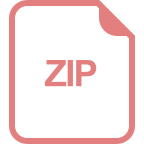