scatter3(sample_data(:,1), sample_data(:,2), sample_data(:,3), 10, idx, 'filled')解释
时间: 2024-06-05 20:07:43 浏览: 18
这行代码使用 scatter3 函数在三维空间中绘制散点图。它的参数含义如下:
- sample_data(:,1):表示取样数据的第一列,即 x 坐标;
- sample_data(:,2):表示取样数据的第二列,即 y 坐标;
- sample_data(:,3):表示取样数据的第三列,即 z 坐标;
- 10:表示每个散点的大小;
- idx:表示每个散点的颜色,这里使用的是一个向量,其中每个元素的值表示对应散点的颜色编号;
- 'filled':表示将散点填充。
这行代码的作用是将三维散点数据可视化,并按照给定的颜色编号进行着色。其中,颜色编号可以是任意整数,但相同的编号会使用相同的颜色,不同的编号会使用不同的颜色。
相关问题
import scipy.io as scio import numpy as np from sklearn.decomposition import PCA from sklearn import svm import matplotlib.pyplot as plt import random from sklearn.datasets import make_blobs test_data = scio.loadmat('D:\\python-text\\AllData.mat') train_data = scio.loadmat('D:\\python-text\\label.mat') print(test_data) print(train_data) data2 = np.concatenate((test_data['B021FFT0'], test_data['IR007FFT0']), axis=0) data3 = train_data['label'] print(data2) print(data3) # print(type(data3)) # print(data4) # print(type(data4)) data2 = data2.tolist() data2 = random.sample(data2, 200) data2 = np.array(data2) data3 = data3.tolist() data3 = random.sample(data3, 200) data3 = np.array(data3) # data4,data3= make_blobs(random_state=6) print(data2) print(data3) # print(type(data3)) # 创建一个高斯内核的支持向量机模型 clf = svm.SVC(kernel='rbf', C=1000) clf.fit(data2,data3.reshape(-1)) pca = PCA(n_components=2) # 加载PCA算法,设置降维后主成分数目为2 pca.fit(data2) # 对样本进行降维 data4 = pca.transform(data2) # 以散点图的形式把数据画出来 plt.scatter(data4[:, 0], data4[:, 1], c=data3,s=30, cmap=plt.cm.Paired) # 建立图像坐标 axis = plt.gca() xlim = axis.get_xlim() ylim = axis.get_ylim() # 生成两个等差数列 xx = np.linspace(xlim[0], xlim[1], 30) yy = np.linspace(ylim[0], ylim[1], 30) # print("xx:", xx) # print("yy:", yy) # 生成一个由xx和yy组成的网格 X, Y = np.meshgrid(xx, yy) # print("X:", X) # print("Y:", Y) # 将网格展平成一个二维数组xy xy = np.vstack([X.ravel(), Y.ravel()]).T Z = clf.decision_function(xy).reshape(X.shape) # 画出分界线 axis.contour(X, Y, Z, colors='k', levels=[-1, 0, 1], alpha=0.5, linestyles=['--', '-', '--']) axis.scatter(clf.support_vectors_[:, 0], clf.support_vectors_[:, 1], s=100,linewidth=1, facecolors='none') plt.show()修改一下错误
根据你提供的代码,可能存在以下几个问题:
1. 在导入make_blobs时没有使用,可以删除或注释掉相关代码:
```
from sklearn.datasets import make_blobs
# ...
# data4,data3= make_blobs(random_state=6)
```
2. 数据集`test_data`和`train_data`中的数据和标签可能没有对应,可以通过打印数据集的shape比对数据数量是否一致。
3. 对数据集进行随机采样时,可能会导致数据和标签对应错误,可以使用numpy的shuffle函数进行随机打乱。
4. 在训练SVM模型时,数据集的标签需要进行reshape操作,以确保标签和数据数量一致。
修改后的代码如下:
```
import scipy.io as scio
import numpy as np
from sklearn.decomposition import PCA
from sklearn import svm
import matplotlib.pyplot as plt
from sklearn.datasets import make_blobs
test_data = scio.loadmat('D:\\python-text\\AllData.mat')
train_data = scio.loadmat('D:\\python-text\\label.mat')
data2 = np.concatenate((test_data['B021FFT0'], test_data['IR007FFT0']), axis=0)
data3 = train_data['label'].reshape(-1)
# 随机打乱数据集
indices = np.arange(data2.shape[0])
np.random.shuffle(indices)
data2 = data2[indices]
data3 = data3[indices]
# 选择前200个数据作为训练集
data2 = data2[:200]
data3 = data3[:200]
# 创建一个高斯内核的支持向量机模型
clf = svm.SVC(kernel='rbf', C=1000)
clf.fit(data2, data3)
pca = PCA(n_components=2)
pca.fit(data2)
data4 = pca.transform(data2)
plt.scatter(data4[:, 0], data4[:, 1], c=data3, s=30, cmap=plt.cm.Paired)
axis = plt.gca()
xlim = axis.get_xlim()
ylim = axis.get_ylim()
xx = np.linspace(xlim[0], xlim[1], 30)
yy = np.linspace(ylim[0], ylim[1], 30)
X, Y = np.meshgrid(xx, yy)
xy = np.vstack([X.ravel(), Y.ravel()]).T
Z = clf.decision_function(xy).reshape(X.shape)
axis.contour(X, Y, Z, colors='k', levels=[-1, 0, 1], alpha=0.5, linestyles=['--', '-', '--'])
axis.scatter(clf.support_vectors_[:, 0], clf.support_vectors_[:, 1], s=100, linewidth=1, facecolors='none')
plt.show()
```
希望这些修改能够帮助你解决问题。
ef m_step(self, posterior): """Maximization step in EM algorithm, use last time posterior p(z|x) to calculate params gratitude. Args: posterior: [n_sample, n_class] p(z=i | x_i, \theta_t) Return: Each class param's gratitude in current time step grad_class_prob: scatter of class j grad_mus: [,dim] jth class mus grad_sigma: [, dim, dim] jth class sigma """ for cls in range(self.n_class): ## class_prob gratitudes grad_class_prob = posterior[:, cls].sum() / self.n_sample ## mu_j <- (\sum_i p(z_j|x_i) * x_i) / sum_i p(z_j |x_i) grad_mus = np.zeros(self.n_dim) for ind in range(self.n_sample): grad_mus += posterior[ind, cls] * self.data[ind, :] grad_mus /= posterior[:, cls].sum() ## sigma_j <- (\sum_i p(z_j|x_i) * (x_i - \mu_j)^2) / sum_i p(z_j |x_i) grad_sigma = np.zeros((self.n_dim, self.n_dim)) for ind in range(self.n_sample): grad_sigma += posterior[ind, cls] * \ np.dot((self.data[ind, :] - self.mus[cls]), self.data[ind, :] - self.mus[cls].T) grad_sigma /= posterior[:, cls].sum() yield grad_class_prob, grad_mus, grad_sigma 这段代码的作用
这段代码是高斯混合模型的EM算法中的M步骤,即最大化步骤,用上一步得到的后验概率p(z|x)来计算模型的参数(即类别概率、均值和协方差矩阵)的梯度。
具体来说,这段代码中的posterior是一个n_samples x n_components的矩阵,表示每个样本属于每个聚类的后验概率。grad_class_prob、grad_mus和grad_sigma分别表示类别概率、均值和协方差矩阵的梯度,通过yield关键字返回结果。
代码的第一个循环计算类别概率的梯度,即将某个聚类中所有样本的后验概率相加后除以样本总数,得到该聚类的类别概率的梯度。
代码的第二个循环计算均值的梯度,即将某个聚类中所有样本的后验概率乘以该样本的特征向量相加,然后除以该聚类所有样本的后验概率之和,得到该聚类的均值的梯度。
代码的第三个循环计算协方差矩阵的梯度,即将某个聚类中所有样本的后验概率乘以该样本与该聚类均值之差的矩阵相乘,然后加权求和,最后除以该聚类所有样本的后验概率之和,得到该聚类的协方差矩阵的梯度。
最后,通过yield返回三个梯度,用于更新EM算法中的参数。
相关推荐
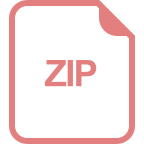
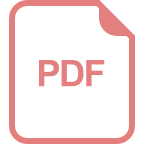
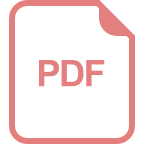












